Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial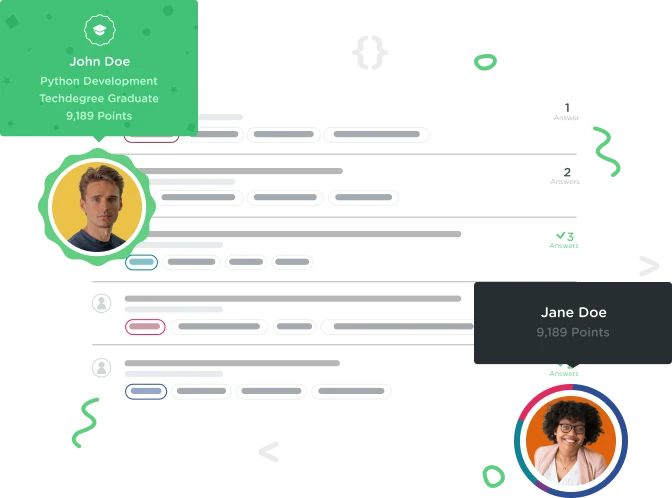
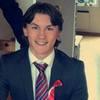
Christopher Gjelten
2,305 PointsIs this a proper way of doing it? It works, but is this something I should do?
var adjective = prompt("Add a adjective");
var verb = prompt("Add a verb");
var noun = prompt("Add a noun");
alert("Ready to see your story");
document.write('The mans hat is ' + adjective + ", he is " + verb + ". He lives on " + noun + ".");
It works but is it something I should do?
2 Answers

Tim Knight
28,888 PointsWhile this isn't something that was covered in the video I think it's helpful to point out that in modern JavaScript (ES6) you also have the option of using string literals when you work with code like this. With string literals (or template literals) you could simplify the output of the string like this:
var adjective = prompt("Type an adjective: ");
var verb = prompt("Type a verb: ");
var noun = prompt("Type a noun: ");
var message = `There once was a ${adjective} programmer who wanted to use JavaScrit to ${verb} the ${noun}`;
alert("Are you ready?");
document.write(message);
Using string literals you'd use back-ticks instead of quotes and anywhere you wanted to output ( interpolate) the variable within the string you'd just use ${variable} as your syntax. Since it's modern JS sometimes older browsers won't understand this newer style (and would require a build step to transpile to an older syntax), but to answer your question in terms of practice this would be the way I'd approach it in an everyday problem.
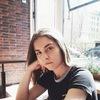
Alissa Kuzina
5,835 Pointsvar adjective = prompt("Type an adjective: ");
var verb = prompt("Type a verb: ");
var noun = prompt("Type a noun: ");
var message = "There once was a ";
message += adjective + " programmer who wanted to use JavaScrit to ";
message += verb + " the " + noun;
alert("Are you ready?");
document.write(message);
This is my version of task. I actually don't think that there IS a proper way to do it. Because as you can see you can concatenate your string in different parts of your code. Your and mine both of them work)
You can also change the view of your HTML and add HTML tags like this
var adjective = prompt("Type an adjective: ");
var verb = prompt("Type a verb: ");
var noun = prompt("Type a noun: ");
var message = "<h2>There once was a ";
message += adjective + " programmer who wanted to use JavaScrit to ";
message += verb + " the " + noun + ".</h2>";
alert("Are you ready?");
document.write(message);
It could be not h2 but <h3>,<h4>,<p> and so on as they have different meaning and style. For example, h1 has the biggest font size by default.
Robert Berek
3,036 PointsRobert Berek
3,036 PointsI am following this, because I did a similar thing.