Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial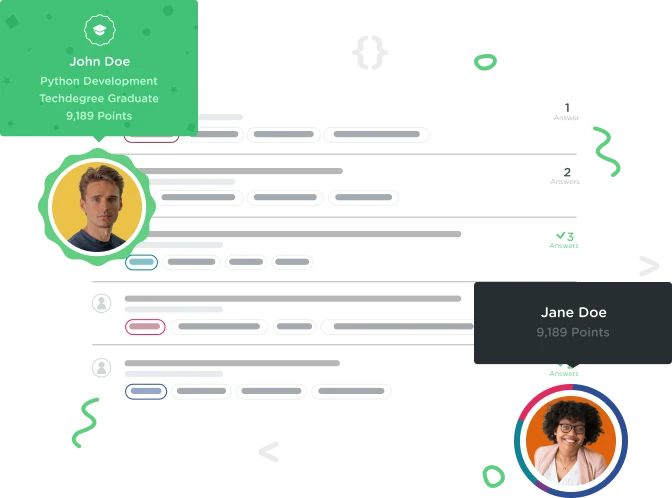

Gareth Welton
2,292 PointsIs this an acceptable code choice here?
namespace TreehouseDefense
{
class Invader
{
private readonly Path _path;
private int _pathStep = 0;
public MapLocation Location => _path.GetLocationAt(_pathStep);
public int Health { get; private set; } = 2;
public bool HasScored { get { return _pathStep >= _path.Length;}}
public bool IsNeutralized { get { return Health <=0;}}
public Invader(Path path)
{
_path = path;
}
public void Move() => _pathStep += 1;
public void DecreaseHealth(int factor)
{
Health -= factor;
}
}
}
1 Answer
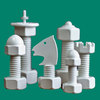
Steven Parker
231,275 PointsI'd say anything that works and is readable is "acceptable". But you do have a curious mix of computed properties and expression-bodied members. It might be better just as a general practice to use one form consistently.
For example:
// since you have this:
public MapLocation Location => _path.GetLocationAt(_pathStep);
// I might expect to also see these:
public bool HasScored => _pathStep >= _path.Length;
public bool IsNeutralized => Health <= 0;
Gareth Welton
2,292 PointsGareth Welton
2,292 PointsThanks Steven, great feedback!