Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial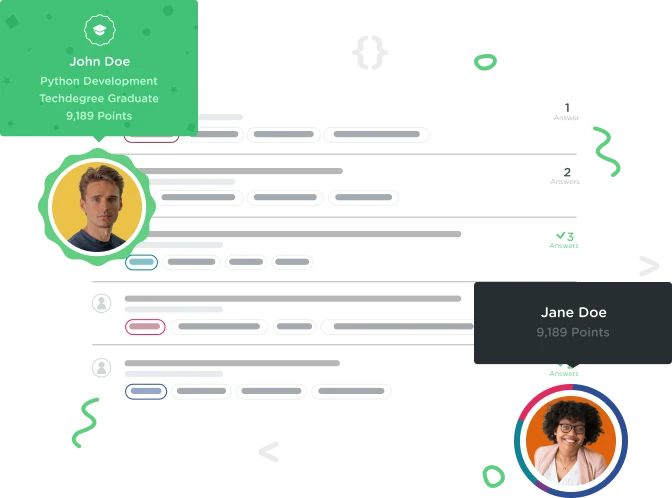

Nathan Holzinger
5,688 PointsIs this asking me to get the first 140 characters of mText? Or everything after the 140th character?
This is from Challenge Task 2 of 2 from Stage 3 of Creating the MVP.
The question is: "Add a new method that returns the remaining characters available, based on the length of what is currently stored in mText."
I keep getting error code: "Whoops: java.lang.ClassCastException: java.lang.String cannot be cast to java.lang.Integer" and I want to be sure I'm actually trying to solve the correct problem.
The error occurs when I "check work" not when I "preview"
I read the question as "return a string of all of the extra characters after the 140th character". Am I on the right track and just have bad code?
public class Tweet {
public static final int MAX_CHAR = 140;
private String mText;
public Tweet(String text) {
mText = text;
}
public String getText() {
return mText;
}
public String getExtraText() {
String extraText = "";
if (mText.length() > MAX_CHAR) {
for (int i=MAX_CHAR; i<mText.length(); i++) {
extraText += mText.charAt(i);
}
}
return extraText;
}
}
3 Answers

Ken Alger
Treehouse TeacherNathan;
Welcome to Treehouse!
Task 2
**Add a new method
that return
s the remaining characters available, based on the length
of what is currently stored in mText
.
Let's break this task down given the code after we finished Task 1 of:
public class Tweet {
public static final int MAX_CHAR = 140;
private String mText;
public Tweet(String text) {
mText = text;
}
public String getText() {
return mText;
}
}
We need to create a method
, which you did, I'm going to call mine charactersLeft
, doesn't much matter, just what I choose. We need to return
the number of remaining characters available, so our return type in this case should be an int
. Our method then would be a public int charactersLeft() {}
.
Now, what do we need our function to do? We need it to calculate how many characters left (from our MAX_CHAR of 140) we have. That value, as you know, can be calculated with mText.length()
. So, to get how many characters we have left we could do something like:
public int charactersLeft() {
int charsLeft = MAX_CHAR - mText.length();
return charsLeft;
}
Does that make any sense?
Happy coding,
Ken

Matt Bloomer
10,608 PointsI have a quick question. Why is that when you are declaring the method you use charactersLeft, and when you call it in the code block you use charsLeft?

Janet Villarreal
3,490 Pointsthat's the variable name
int charsLeft

Gonzalo Nunez
Courses Plus Student 5,064 PointscharactersLeft is the name of the method only, you´ll use this to call the method.
The charsLeft is the variable he created to store result of ( MAX_CHARS - mText.lenght());

Daniel Anderson
1,622 PointsTY it helped!
Nathan Holzinger
5,688 PointsNathan Holzinger
5,688 PointsThanks Ken!
I misunderstood the question. I thought we were to assume that we'd be given a string that was over 140 characters and then we were supposed to return the all the extra characters that would be left over after the text was truncated to 140 characters.
Your solution worked, thanks for your help!
Lloyd McCluskey
11,073 PointsLloyd McCluskey
11,073 PointsThank you for spelling it out for me!
Awais jamil
Java Web Development Techdegree Student 4,742 PointsAwais jamil
Java Web Development Techdegree Student 4,742 Pointsthanks for explanation