Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial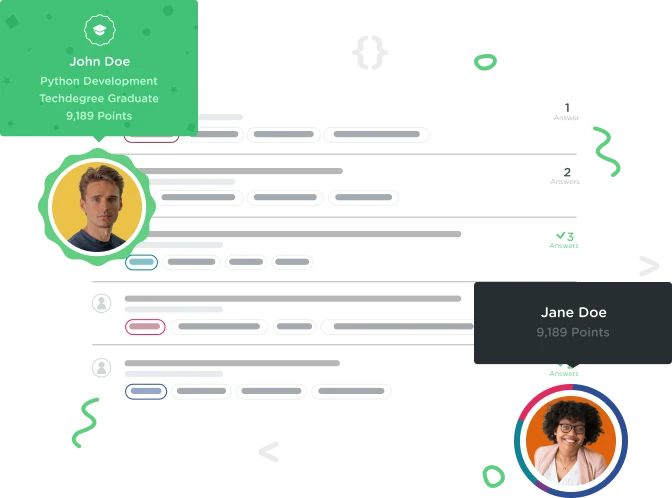

Martin Enriquez
7,577 PointsIs this checking for errors properly? I have reproduced the output in a different environment
I am able to reproduce the required output in a different environment and even in the REPL. The challenge says "Task 1 is no longer passing" and Task 1 is to import the random library. Can you provide an insight into what is causing this challenge to fail?
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start != 0:
number = random.randint(1,99)
if even_odd(number) == True:
print "{number} is even".format(number)
else:
print "{number} is odd".format(number)
start -= 1
2 Answers

Charlie Gallentine
12,092 PointsSo in your code, when you call the format string, you do not need to enter 'number' between the curly braces. Additionally, you are missing parentheses around the contents of the print function. The .format(number) takes care of filling the braces for you, along with parentheses:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start != 0:
number = random.randint(1,99)
if even_odd(number) == True:
print "{} is even".format(number)
else:
print "{} is odd".format(number)
start -= 1
While this isn't strictly applicable to this challenge (the code will still pass), a piece of advice for checking if a value is falsey, instead of checking if it isn't zero, simply check if it exists at all within python's definitions of truthy and falsey:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
number = random.randint(1,99)
if even_odd(number) == True:
print "{} is even".format(number)
else:
print "{} is odd".format(number)
start -= 1
Because 0 is a falsey value, the loop will stop once start == 0 as that will make start cease to be a truthy.
In terms of TreeHouse error messages, the 'task # is no longer passing' error message is usually an indicator of a syntax error rather than any effect which may be acting on the prior tasks which took me a bit to realize.
Hope that helps!

Martin Enriquez
7,577 PointsThanks! Indeed it was a syntax error. I took the var names out of the curly brackets and wrapped the 'messages' around parenthesis in the print function and it passed.