Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial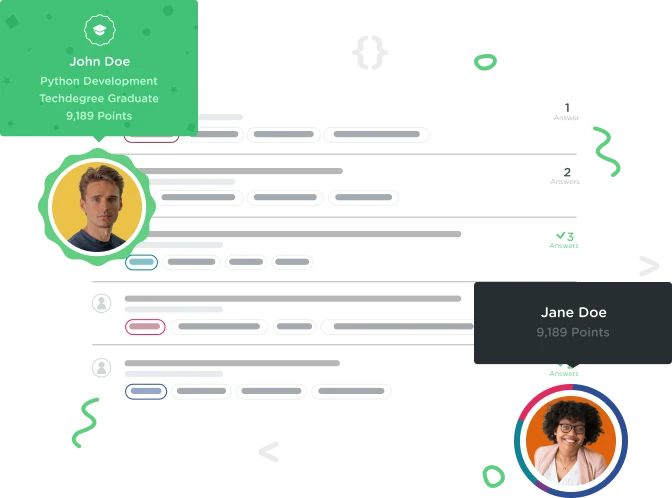

Jonathan Söder
7,428 PointsIs this code good or could I have done something better or more effective?
I wanted the user to be able to pass in information rather than hard code two values in the program so I ended up with this instead:
My questions are, is this code passable? Could I have done something different or more effective? Is it hard to read, too much clutter? Thanks
/*****************/
/****Functions****/
/*****************/
function upperLower(){
//Prompt user for values
a = parseInt(prompt ("Type the lowest possible value") );
b = parseInt(prompt ("Type the highest possible value") );
//Store value in roll
roll = Math.floor(Math.random() * (b - a + 1)) + a;
//Inform user of their choice
document.write("<p>You chose: <br>" +
"lowest number: " + a + "<br>" +
"highest number: " + b + "<br>" + "</p>");
//Return value
return roll;
}
/************/
/****main****/
/************/
//Call function
upperLower();
//Show result
document.write("<p>You rolled a " + roll + ".</p>");
3 Answers
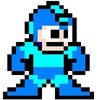
Robert Richey
Courses Plus Student 16,352 PointsHi Jonathan,
You're doing great :). My C++ college professor preached to us that working, sub-optimized code is always better than broken, optimized code.
With that being said, there is almost always room for improvement depending on what you choose to care about. For example, and this is just my opinion, when your code is well written - it is self documenting. Use comments very sparingly for sections that are not intuitive.
Also, consider validating user input. There are 3 important rules regarding user input:
- never trust user input
- never trust user input
- never trust user input! :)
What if they enter 10 for the lowest value and 1 for the highest? What if they enter letters? What if they don't enter anything at all? ... yeah, validating user input is super fun.
Finally, instead of making a variable to hold the value being returned, just return the value.
With these changes in mind, here is my take on your code - except I'm not doing full input validation, just swapping values if min > max.
function upperLower(){
var min = parseInt(prompt("Type the lowest possible value"));
var max = parseInt(prompt("Type the highest possible value"));
if (min > max) {
var temp = min;
min = max;
max = temp;
}
document.write("<p>You chose: <br>" +
"lowest number: " + min + "<br>" +
"highest number: " + max + "<br>" + "</p>");
return Math.floor(Math.random() * (max - min + 1)) + min;
}
document.write("<p>You rolled a " + upperLower() + ".</p>");

Jonathan Söder
7,428 Points"when your code is well written - it is self documenting. Use comments very sparingly for sections that are not intuitive." right, I'll try to remember it the next time!
"never trust user input never trust user input never trust user input! :)" This is something that's so blatantly obvious when hearing it but something that I've never really thought about. I usually go around thinking how to build stuff but never how to keep stuff from not breaking. A lot of things I want to create is heavy on the user input department so this is something I'll probably put on a note and attach to my monitor.
Thank you so much for your input. I definitely learned something from this and I'll remember to take it easy on the comments :=)

Charles Smith
7,575 PointsI would also note that while Robert didn't mention it, he chose to give the variables more descriptive variable names. This doesn't change the function of the program, but it does make it more readable and more easy to debug. Though it's my inclination to do exactly as you did, I force myself to give more descriptive variable names as though someone else were going to have to read and maintain it. The other thing to keep in mind is that you don't save time programming by reducing typing, you do save time programming by make your programs readable and easier to debug.
Jim Withington
12,025 PointsJim Withington
12,025 PointsThe next video in the series actually addresses this.
https://teamtreehouse.com/library/javascript-basics-2/creating-reusable-code-with-functions/random-number-challenge-part-ii