Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial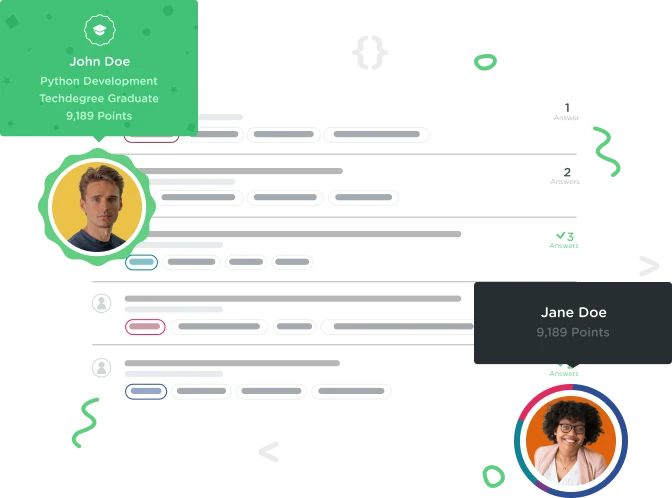

keith frazier
Python Development Techdegree Student 5,771 PointsIs this code stinky?
I had such a different approach that I am wondering if my code is stinky or informal somehow and I am just not seeing it. Here is my code:
# TODO Create an empty list to maintain the player names
player_names = []
# TODO Ask the user if they'd like to add players to the list.
player_number = 1
while True:
add_to_list = input("Do you want to add a player to the list? (Yes\\No)" )
if add_to_list.lower() == 'yes':
# If the user answers "Yes", let them type in a name and add it to the list.
player_name = input("Enter the name of the player you would like to add to the team:" )
player_names.append(player_name)
continue
# If the user answers "No", print out the team 'roster'
elif add_to_list.lower() == 'no':
# TODO print the number of players on the team
print("There are {} players on the team.".format(len(player_names)))
# TODO Print the player number and the player name
for player in player_names:
print("Player {}: {}".format(player_number, player))
# The player number should start at the number one
player_number = player_number + 1
# TODO Select a goalkeeper from the above roster
goal_keeper = 1 - (int(input("Please select the goal keeper by selecting the player number. (1-{})".format(len(player_names)))))
break
# TODO Print the goal keeper's name
# Remember that lists use a zero based index
print("Great!! The goalkeeper for the game will be {}".format(str(player_names[goal_keeper])))
I was even going to go further and make a function to print the names then call it in the for loop, but I figured I was probably already way off base..
I am seeing now that the last line of code didn't need to coerce the string type, that is the kind of stank I feel is lingering around the rest of the script...
1 Answer
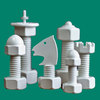
Steven Parker
231,275 PointsA few minor things stand out at first glance:
- a "continue" is not needed when there is no following code that might be done in the loop
- the goal keeper value should be made by subtracting 1 from the input (not vice-versa)
- the outer parentheses around the "int" function are not needed
- since "player_number" is not used elsewhere, it could be created for the loop with "enumerate"
And example of the last item:
for player_number, player in enumerate(player_names, 1):
print("Player {}: {}".format(player_number, player))