Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial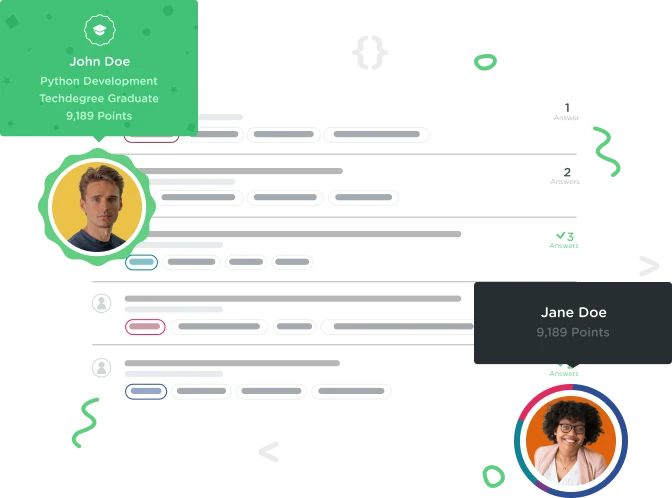

Daniel Kim
Courses Plus Student 526 PointsIs this considered wrong or messy code?
The video had the if statement within the function. Just outta curiosity I tried to use the if statement outside of the function and below the input and it worked. Is this considered wrong or improper formatting?
import math
def split_check(total, number_of_people):
return math.ceil(total / number_of_people)
try:
total = float(input("What is the total? "))
number_of_people = int(input("How man people? "))
if number_of_people <=1:
raise ValueError("More than 1 person is required to split a check")
amount_due = split_check(total, number_of_people)
except ValueError as err:
print("Oh no! That's not a valid value. Try again")
print("{}".format(err))
else:
print("Each person owes ${}".format(amount_due))
1 Answer
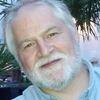
Jeff Muday
Treehouse Moderator 28,720 PointsYour code is easy to read with nicely named variables, good job! You also used a well named function name, split_check()
It is very clear what you are intending to do. This will serve you well on your journey to become a software developer.
Your code is clean, but the one "gotcha" is nesting a raise
inside a try except
block. While it is syntactically valid, it can have unpredictable results. In your case, you get it right!
Personally, if I am presented with this kind of task where a user will give unpredictable input I like to embed the input into an event loop. I also like to encapsulate the input to a particular type like the get_number()
function I show below.
Good luck with Python! There are so many programs to write and not enough hours in the day!!
import math
def split_check(total, number_of_people):
return math.ceil(total / number_of_people)
def get_number(prompt):
import sys
while True:
# event loop continues to ask for a number until it succeeds or
# user replies with a "quit" or a "q"
num = input(prompt)
if num.upper().startswith('Q'):
print("exiting program")
sys.exit(0)
try:
value = float(num)
return value
except:
print("Oh no! That's not a valid value. Try again")
while True:
# main event loop
total = get_number("What is the total of the check? (or quit) ")
number_of_people = int(get_number("Number of ways to split? (or quit)"))
if number_of_people <=1:
print("More than 1 person is required to split a check")
else:
amount_due = split_check(total, number_of_people)
print("Each person owes ${}".format(amount_due))
Test it out.
What is the total of the check? (or quit) Yikes shouldn't have ordered dessert!
Oh no! That's not a valid value. Try again
What is the total of the check? (or quit) 88.52
Number of ways to split? (or quit)3
Each person owes $30
What is the total of the check? (or quit) 102.50
Number of ways to split? (or quit)-1
More than 1 person is required to split a check
What is the total of the check? (or quit) 102.50
Number of ways to split? (or quit)5
Each person owes $21
What is the total of the check? (or quit) quit
exiting program