Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial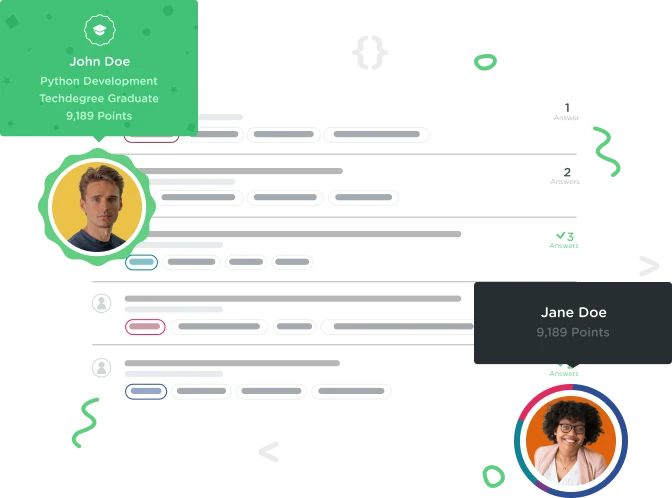

Kishan S
15,410 PointsIs this Correct? Any simpler alternative using while statement?
Any Thoughts?
var temperatures = [100,90,99,80,70,65,30,10];
var l = temperatures.length;
var i = 0;
while(l >= 0) {
console.log(temperatures[i]);
l-=1;
i+=1;
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
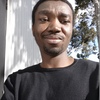
Andrews Gyamfi
Courses Plus Student 15,658 PointsThere is an Off-by-one error in your loop. This is how your code currently runs (I actually used Codepen.io to add some testing/debugging the code)
Modified Code:
var temperatures = [100,90,99,80,70,65,30,10];
var l = temperatures.length;
var i = 0;
while(l >= 0) {
document.write(temperatures[i] + ", ");
/* debugging code*/
document.write("length: " + l + " | " + "index: " + i + "<br/>");
/* loop counter increments */
l-=1;
i+=1;
}
Output:
100, length: 8 | index: 0
90, length: 7 | index: 1
99, length: 6 | index: 2
80, length: 5 | index: 3
70, length: 4 | index: 4
65, length: 3 | index: 5
30, length: 2 | index: 6
10, length: 1 | index: 7
undefined, length: 0 | index: 8
An array index starts from 0 to its length minus 1. In your code, you used the comparison
while(l >= 0)
However, as per the above output, when your length (l variable) has been reduced to 0 your index (i variable) would have incremented to 8 which is 'undefined' or null as your array only has eight elements.
Solution: change while(l>=) to while(l > ) i.e remove '='
var temperatures = [100,90,99,80,70,65,30,10];
var l = temperatures.length;
var i = 0;
while(l > 0) {
document.write(temperatures[i] + ", ");
/* debugging code*/
document.write("length: " + l + " | " + "index: " + i + "<br/>");
/* loop counter increments */
l-=1;
i+=1;
}
Output:
100, length: 8 | index: 0
90, length: 7 | index: 1
99, length: 6 | index: 2
80, length: 5 | index: 3
70, length: 4 | index: 4
65, length: 3 | index: 5
30, length: 2 | index: 6
10, length: 1 | index: 7
Hope this helps :) Cheers!

Rich Donnellan
Treehouse Moderator 27,696 PointsNo, this isn't correct. The l
(length) variable should not be decremented at all.
Check out the for
loop; this does exactly what you're asking for (pun intended).
Provided code rewritten with for
loop:
var temperatures = [100, 90, 99, 80, 70, 65, 30, 10];
for (var i = 0; i < temperatures.length; i++) {
console.log(temperatures[i]);
}

Kishan S
15,410 PointsYes I Know that. but how to use while loop in that?
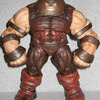
Jacie Fortune
8,969 PointsYou shouldn't decrement l as the length of the array does not change. Here it is using a while loop. <code> var temperatures = [100, 90, 99, 80, 70, 65, 30, 10]; var i = 0; while (i < temperatures.length) { console.log(temperatures[i]); i += 1; } </code>

Kishan S
15,410 PointsThank you!