Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial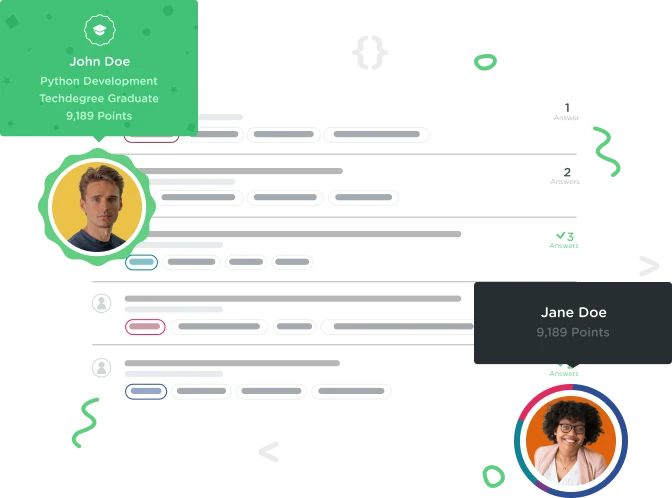
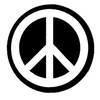
john larson
16,594 Pointsis this function built into js
function print(message) {}...I'm trying to make sense of a number of things in the lesson and this is one of them. I don't recall it being mentioned that this particular function is built in, but I get the concept that some things ARE built in. It confuses me because there is no "message" outside this function that it would be relating to.
1 Answer
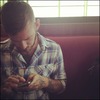
Erik McClintock
45,783 PointsJohn,
If you're referring to the following code:
function print( message ) {
document.write( '<p>' + message + '</p>' );
}
Then no, that function is not built in, but is a custom function that was written in that script. There are a number of things "built in" to JavaScript, and there does happen to be a native print()
method on the window
object, but this is not that.
The "message" in the parenthesis of the function is what's known as a "parameter", which is a name listed in a function definition to indicate that the function expects to be passed an argument when it is called later on in your script. Think of it kind of like a variable: it will hold a value to be used later. "Message" is not defined elsewhere, but rather is simply what the teacher has chosen to call the parameter. He could call it anything he wanted! It could be called "bananaWaffle" if he so chose. That is simply a placeholder name for what value will be passed in as an argument when that function is used later for practical purposes.
Let's look an example of defining and then calling another function to illustrate this point:
function addTwoNumbers( numOne, numTwo ) {
var sum = numOne + numTwo;
return sum;
}
console.log( addTwoNumbers( 5, 10 ) );
In the above code, we first define a function, choosing to call it "addTwoNumbers". We then define that our function will accept/expect two parameters, and we've decided to name those parameters "numOne" and "numTwo" (though, again, we could've named them anything we wanted!). Inside our function, we instruct it to create another new variable, choosing to call it "sum", and setting the value of that "sum" variable equal to the result of adding the values that were passed into the function, which are stored in our "numOne" and "numTwo" variables (once the function is called later in our script, of course!). We then use the keyword "return" to do just that - return the value stored in our "sum" variable! That means that, when we call this "addTwoNumbers" function elsewhere in our script, it will take whatever two numbers we gave it, and return the result of those two numbers added together.
After our function, we write a simple console.log statement (which is a "built-in" JavaScript object and method), and inside call our function "addTwoNumbers", passing in (you guessed it!) two numbers (any two numbers we want!) for our arguments, in this case, 5 and 10. Our function will run, doing what it's meant to do (adding the two numbers given to it, and returning that sum), and then our console.log statement will do what it is meant to do: log that result to the console (which, in this case, will be the number 15)!
Hope this helps to clarify some things!
Erik