Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial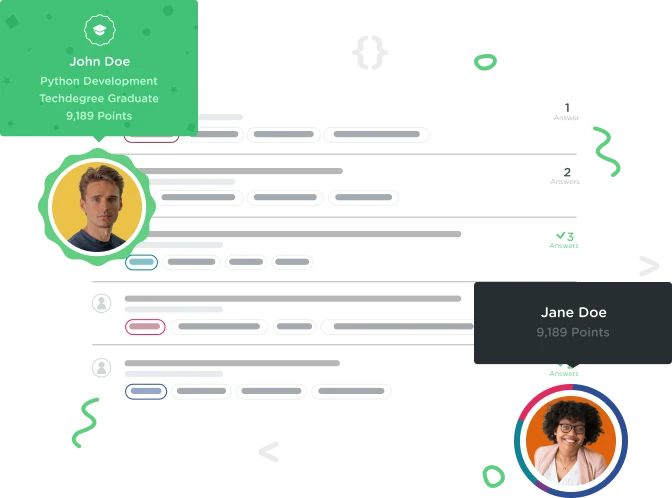
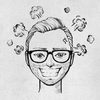
Ronny Gudvangen
4,685 PointsIs this good practise?
Pretty new with JS, so I wonder if this is good practise? I would like to expand the script with some animation/effects, but it's now working correctly.
It edits the visibility of to divs placed on top of each other. The reason for not using display is that I need it to take up space in the browser.
$(document).ready(function flipPanels() {
var $element = '.flip-container';
$($element).click(function() {
var $front = $(this).children('.front');
var $back = $(this).children('.back');
if( $front.css('visibility') == 'visible' ) {
$(this).children('.front').css('visibility', 'hidden');
$(this).children('.back').css('visibility', 'visible');
} else {
$(this).children('.front').css('visibility', 'visible');
$(this).children('.back').css('visibility', 'hidden');
};
});
});
6 Answers

Chris Malcolm
2,909 Pointsyou can also use opacity 0 or 1. I personally would simplify it and put more logic into css.do this by defining your element .flip_container with another class to toggle another operation.
.flip-container .front{opacity: 1}
.flip-container .back{opacity: 0}
.flip-container.flip .front{opacity: 0}
.flip-container.flip .back{opacity: 1}
$($element).click(function() {
if ($(this).hasClass("flip"))
$(this).removeClass("flip");
else
$(this).addClass("flip");
});
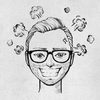
Ronny Gudvangen
4,685 PointsThanks, Chris!
But if I want to fade the opacity from 1 to 0, can this easily be applied by adding and removing classes?
Perhaps domething like this.
$(this).removeClass("flip").fadeIn('fast');
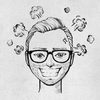
Ronny Gudvangen
4,685 PointsAllright. I now have this, which works fine:
$(document).ready(function flipPanels() {
var $element = '.flip-container';
$($element).click(function() {
var $front = $(this).children('.front');
var $back = $(this).children('.back');
if( $front.css('opacity') == '1' ) {
$(this).children('.front').fadeTo("slow", 0);
$(this).children('.back').fadeTo("slow", 1);
} else {
$(this).children('.front').fadeTo("slow", 1);
$(this).children('.back').fadeTo("slow", 0);
};
});
});
But I find this to be a simpler solution. Only problem is adding a fadein/out effect to when the classes are added. The classes change the opacity.
$(document).ready(function flipPanels() {
var $clickElement = '.flip-container';
$($clickElement).click(function() {
var $flipElements = $(this).children('.front, .back');
if( $flipElements.hasClass("flip") ) {
$flipElements.removeClass("flip");
} else {
$flipElements.addClass("flip");
};
});
});

Chris Malcolm
2,909 Pointsthis is perfect time to use css transitions . below will create a .5 second fade for opacity attribute of your front and back any time you change the opacity.
.front,
.back{
transition: opacity .5s ease-in-out;
-moz-transition: opacity .5s ease-in-out;
-webkit-transition: opacity .5s ease-in-out;
}
Check out an example of this here: http://codepen.io/anon/pen/CxsIJ
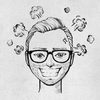
Ronny Gudvangen
4,685 PointsAllrighy! Solved this way:
```$(document).ready(function flipPanels() { var $clickElement = '.flip-container';
$($clickElement).click(function() {
var $flipElements = $(this).children('.front, .back');
$flipElements.toggleClass("flip-opacity");
});
});
Also included transition to the CSS. Jquery toggleClass proved to be a good shorthand instead of my IF statement.

Chris Malcolm
2,909 Pointsyup toggleClass is even better! great job, much cleaner now. if you wanna take it to the limit, instead of declaring a variable, you can chain this.
$(this).children(".front, .back").toggleClass("flip-opacity");
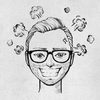
Ronny Gudvangen
4,685 PointsGreat! Thanks