Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial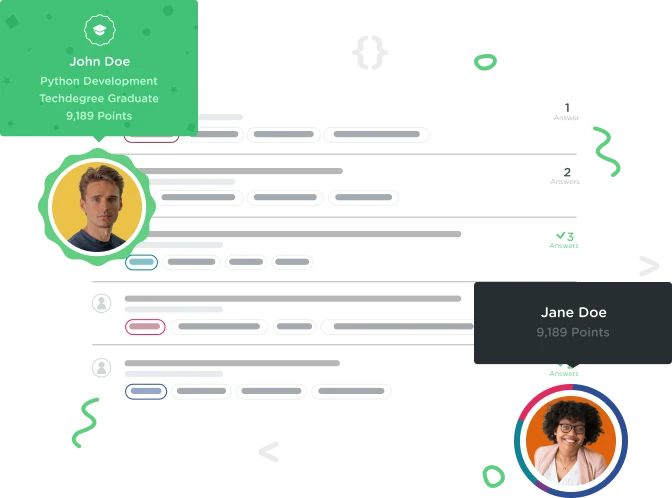

Mason Barton
Full Stack JavaScript Techdegree Student 5,656 PointsIs this method fine?
function getRandomNumber( lower, upper ) { if ( isNan = true) { throw new Error('NOT A NUMBER'); }
I did this and got the error that I needed to get, but he mentioned needing to use ||, which I didnt do, nor could I figure out how
1 Answer

Eric Butler
33,512 PointsUnfortunately, no. Copying your function into multiple lines to see it better:
function getRandomNumber( lower, upper ) {
if ( isNan = true) {
throw new Error('NOT A NUMBER');
}
Several things here:
- You haven't closed your function with a curly brace. Hopefully this is just a copying omission.
- You're not using either argument (
lower
andupper
) you pass into the function. If you don't need them, don't pass them in. - This function will always throw a NOT A NUMBER error, because your
if
statement isn't evaluating anything, it's assigning something. To evaluate something, you have to use double- or triple-equals:a == b
ora === b
. This compares a and b to each other. If you saya = b
, you are assigning the value of b as the value of a, the same way you'd saya = 3
to set the variable's value. Assignments of positive values always return true, so yourif
statement will always run. -
isNaN
first off needs a capital N at the end, and also is not a standalone value, it's a function. You have to call it and you have to pass a value to it:isNaN(3)
orisNaN(a)
. If you do that, it will return true or false. Extra note: since the isNaN() function itself returns true or false, you do not have to compare it totrue
at all in theif
function. You'd usually see this written like so:if ( isNaN(a) ) { /* whatever */ }
sinceisNaN
returning true or false will give theif
condition all it needs to evaluate whether to run or not.
Hope that all makes sense! Cheers.