Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial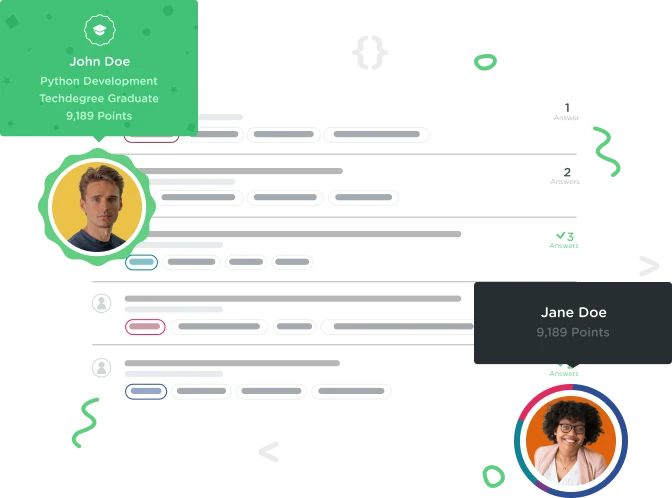

Alejandro Galiano
6,613 PointsIs this solution DRY, understandable and/or efficent? Please have a look.
Hi all! I came up with this solution before watching Dave's and it works just like he wanted (as shown in the previous video). I was wondering if it is efficient, DRY and understandable... Can you find any error or something not very clear? How would you improve it?
var questions = [
["What's the capital of The Netherlands?", "AMSTERDAM"],
["What's the capital of The Bahamas?", "NASSAU"],
["What's the capital of the UK?", "LONDON"],
["What's the capital of the Spain?", "MADRID"],
["What's the capital of the Italy?", "ROME"],
];
var correct = 0;
var questionsRight = [];
var questionsWrong = [];
function print(message) {
document.write(message);
}
function adding (variable) {
variable.push("<li>" + questions[i][0] + "</li>");
}
for (var i = 0; i < questions.length; i++) {
var answers = prompt(questions[i][0]).toUpperCase();
if (answers === questions[i][1]) {
correct += 1;
adding(questionsRight);
} else {
adding(questionsWrong);
}
}
print("<p>You got " + correct + " question\(s\) right of " + questions.length);
var messageRight = "<p style='color:black'>You got these questions correct:</p><ol>" + questionsRight.join("") + "</ol>";
var messageWrong = "<p style='color:black'>You got these questions wrong:</p><ol>" + questionsWrong.join("") + "</ol>";
var messageAllRight = "<p style='color:black'>You got these questions wrong:</p><ul>" + "<li>Wow! No wrong answers. Congratulations!</li>" + "</ul>";
var messageAllWrong = "<p style='color:black'>You got these questions correct:</p><ul>" + "<li>Bummer! No right answers. Sorry.</li>" + "</ul>";
if(correct === questions.length) {
print(messageRight);
print(messageAllRight);
} else if (correct !== 0) {
print(messageRight);
print(messageWrong);
} else {
print(messageAllWrong);
print(messageWrong);
}
Thanks a lot, I appreciate your time.
AG.
1 Answer

Mark VonGyer
21,239 PointsHi. First, your function function print(message) just performs document.write. I wouldn't create a function to do just one function, you may as well use the document.write function later on and pass the message as the parameters.
In the messages section there are two comments that refer to questions right/ wrong but no list is given to the user.
I would change some of the naming conventions to be more clear. eg/ correct ought to be numberCorrect or countCorrect