Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial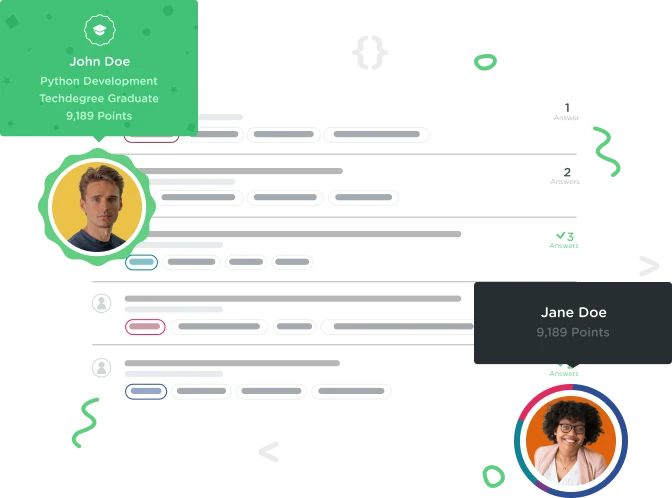

R R
1,570 PointsIs this solution for refactor challenge correct?
I used two functions instead of a loop. Seems to work when I preview. Some questions:
Are these two functions automatically called? I thought I'd have to write:
getRandomNumber();
getColors()
for the functions to run.
Main question: is this a good solution?
var html = '';
var red;
var green;
var blue;
var rgbColor;
var upper = 256;
var randomNumber = getRandomNumber (upper)
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
function getColors () {
red = randomNumber;
green = randomNumber;
blue = randomNumber;
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);

R R
1,570 PointsThanks, Colin.
3 Answers
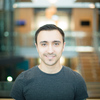
Ed Mendoza
9,652 PointsHi RR,
To answer your first question, you always need to invoke a function, for more reading on the different ways to call a function you can check out this link.
On your second question, I wasn't able to get your code to work inside of a codepen. But after looking at what you wrote, I'm not sure how you are going to loop through each item/color that is created using javascript.
If you wanted to build a function to run instead of just having a loop that executes on page load, you could create something like below.
// Initiate Variables
var html = '',
red,
green,
blue,
rgbColor;
// Set up function
function colorGenerator(itemCount) {
// Loop through number of items
for(i = 0; i < itemCount; i++) {
// Get random colors
red = Math.floor(Math.random() * 256);
green = Math.floor(Math.random() * 256);
blue = Math.floor(Math.random() * 256);
// Create RGB background color
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
// Write new item to html
html += '<div style="background-color:' + rgbColor + '"></div>';
}
// Write html to the document
document.write(html);
}
// Call function with desired number of color items
colorGenerator(10);
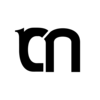
Colin Marshall
32,861 PointsFunctions do not always have to be invoked. Anonymous functions are self-invoked and run when they are declared.
You are correct though in that they need to be invoked in RR's code.
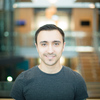
Ed Mendoza
9,652 PointsYou're right Colin, I hadn't thought about using an anonymous function while looking at RR's code. Linking to some relevant material on anonymous functions for OP to check out. link

Iain Simmons
Treehouse Moderator 32,305 PointsColin Marshall, functions do have to be invoked/called if you actually want the code within them to have any effect.
Anonymous functions are just functions without names, and don't run when they are declared (they'd be significantly less useful if that was the case).
You might be thinking of the popular and useful pattern of the Immediately-invoked function expression, which places the anonymous function within a set of parentheses and then is followed by another set of parentheses, which tells the compiler to execute the statement proceeding it.
They don't even have to be anonymous functions to be immediately-invoked, and in some circles, there are recommendations to name all of your functions, just to make everything easier to debug.

R R
1,570 PointsHello again everyone-after being gone for about a year, i finally have been able to get back to this. A little frustrating to not be able to focus on this, but am determined to learn. I revisited this challenge and, without looking at what I wrote originally, I came up with this:
var html = ''; var numberOfCircles = 10;
function getRandomNumber() { var num = Math.floor(Math.random() * 256) + 1; return num; }
function makeCircle(){ var rgbColor = 'rgb(' + getRandomNumber() + ',' + getRandomNumber() + ',' + getRandomNumber() + ')'; html += '<div style="background-color:' + rgbColor + '"></div>'; }
for ( var i = 0; i < numberOfCircles; i += 1 ) { makeCircle(); }
document.write(html);

R R
1,570 PointsThank you for all the replies. Apologies for not expressing my appreciation earlier.
Colin Marshall
32,861 PointsColin Marshall
32,861 PointsHello R R,
I fixed your code. Please see the link on the right titled "Tips for asking questions" for instructions on how to post your code to the forum. Thanks!