Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial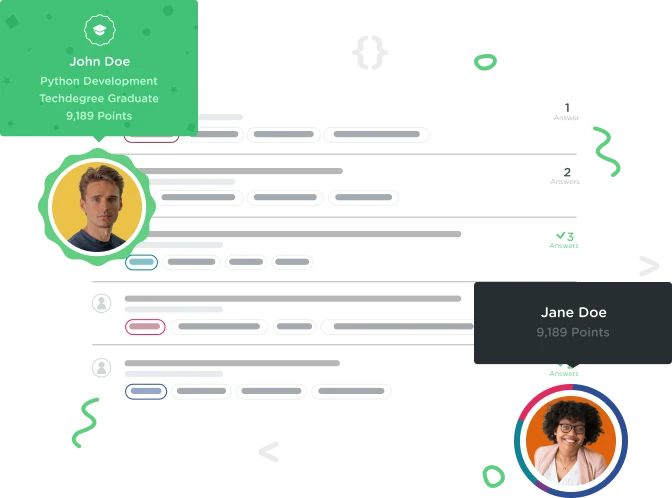

mattcleary2
19,953 Pointsis this task wanting me to use an IF statement? or am i missing the point?
Hey guys,
Just working my way through this, am I supposed to be using an if statement?
Trying to work this one out of my own as much as I can.
Thanks!
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
enum Direction {
case Left
case Right
case Up
case Down
}
class Robot {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: Direction) {
// Enter your code below
}
}
6 Answers

Keli'i Martin
8,227 PointsYou could use an if statement. You could also use a switch statement to solve this.

mattcleary2
19,953 PointsGod damn, yes that worked.
haha thank you!

Keli'i Martin
8,227 PointsGlad it finally worked for you! Keep coding!!

mattcleary2
19,953 PointsOkay, I'm typing a lot of code, but I feel like I'm kinda lost. Am I on the right track?
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
enum Direction {
case Left
case Right
case Up
case Down
}
class Robot {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: Direction) {
// Enter your code below
switch direction{
case Left: location.x -=
case Right: location.x +=
case Up: location.y +=
case Down: location.y +-
}
}
}
let daytype = Robot(Up)
let moveUp = move(direction)

Keli'i Martin
8,227 PointsYou're definitely on the right track. The only issue I see is that you need to use the proper unary increment and decrement operators. -=
and +=
are expecting two operands, ie. location.x += 1
. That is a valid way to fix your code, but you could also use location.x++
, for example. Otherwise, good job!

mattcleary2
19,953 PointsThanks Keli'i,
It's still not passing though.
I just did what you recommended. These are my errors:
swift_lint.swift:43:1: error: expected declaration
^
swift_lint.swift:41:23: error: use of unresolved identifier 'Up'
let direction = Robot(Up)
^
swift_lint.swift:42:14: error: use of unresolved identifier 'move'
let moveUp = move(direction)
^
swift_lint.swift:31:14: error: use of unresolved identifier 'Left'
case Left: location.x -= 1
^
swift_lint.swift:32:14: error: use of unresolved identifier 'Right'
case Right: location.x += 1
^
swift_lint.swift:33:14: error: use of unresolved identifier 'Up'
case Up: location.y += 1
^
swift_lint.swift:34:14: error: use of unresolved identifier 'Down'
case Down: location.y +- 1
^
swift_lint.swift:34:31: error: use of unresolved identifier '+-'
case Down: location.y +- 1
^
swift_lint.swift:34:31: error: operator is not a known binary operator
case Down: location.y +- 1

Keli'i Martin
8,227 PointsOh yeah, I wasn't looking at your code past the switch statement. You are trying to create an instance of your Robot() class, but your initializer doesn't contain any parameters (which it doesn't need for this challenge). Try removing Up
from that. That's first.
Next, you are switching on an enum, so each of your case statements needs to reference the enum correctly. So rather than case Down:
, you need to say case .Down
. The period before Down is important. It is basically a shortcut to saying case Direction.Down
.
Lastly, the correct decrementing binary operator is -=
, not +-
.
Try making those fixes and let me know how it goes.

Keli'i Martin
8,227 PointsOh, one more thing. The move()
function needs to be called from your object. So, daytype.move(.Up)
for example. Second, the function doesn't return anything so you shouldn't be trying to set a constant to its return. In other words let moveUp = daytype.move(.Up)
is invalid. You just need to call daytype.move(.Up)
.

mattcleary2
19,953 PointsSorry, daytype was typo carried over from a previous task. That should say direction. Well I intended it too.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
enum Direction {
case Left
case Right
case Up
case Down
}
class Robot {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: Direction) {
// Enter your code below
switch direction{
case .Left: location.x -= 1
case .Right: location.x += 1
case .Up: location.y += 1
case .Down: location.y -= 1
}
}
}
let direction = Robot()
direction.move(.Up)
The error is now saying 'Expected declaration'?

Keli'i Martin
8,227 PointsHonestly, for passing the challenge, you are not required to declare an instance of Robot
or call move()
. All you need to do is put your switch block into the func move()
function body, and you should be all set.

mattcleary2
19,953 Pointsahhh man, I've taken off those last two lines of code. And it STILL is saying 'expected declaration'

Keli'i Martin
8,227 PointsYou know, the challenge editor can sometimes get into a weird state where no matter what you do, it doesn't build right. Try this: copy the switch block you wrote, reload the challenge page, and then just paste that code into the boilerplate code that you're given. Then try submitting it.