Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial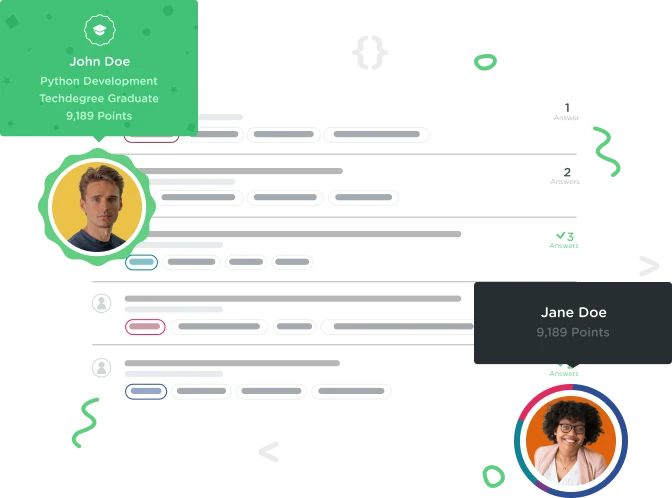
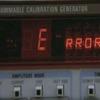
reece
1,975 PointsIs this the most efficient way to write this program?
Hey, Learning Java, I thought it would be cool to improve the "Knock knock" joke program by making it so that the user MUST enter banana or orange otherwise it displays an error. I also replaced console.printf with System.out.println and scanner.
I have used a boolean primitive? to output a string if they enter something other than banana or orange. I'm curious as to what are some other ways of doing this? I just wanna open my mind a bit, I feel as if I am stuck in a box.
import java.util.Scanner;
public class KnockKnock {
public static void main(String[] args) {
Scanner getInput = new Scanner(System.in);
String userAnswer;
boolean otherAnswer;
boolean bananaAnswer;
do {
System.out.println("Knock knock");
System.out.print("Who's there? ");
userAnswer = getInput.nextLine();
bananaAnswer = (userAnswer.equalsIgnoreCase("banana"));
otherAnswer = (userAnswer.equalsIgnoreCase("orange") || userAnswer.equalsIgnoreCase("banana"));
if(!otherAnswer) {
System.out.println("You must answer with: banana or orange");
} else {
System.out.println(userAnswer + " who?");
}
} while(bananaAnswer || !otherAnswer);
System.out.println("Orange you glad to see me?");
}
}
Yes the program in its current state works.
1 Answer
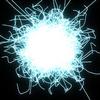
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Reece,
This is certainly a good use of the boolean logic and your || operator which were two pretty big things in this course, so I'd say you did a good job this, you're always going to find more efficient ways to do something especially as you better with coding. With that being said you did an excellent job and showed a good understand of what the course wanted you to know, great job!
as far wanting to open your mind a bit this is a good way to do it what you are already doing, you could create a method that returns a string based on their answer and clean up the code a bit. But that's being nitpicky. Your best bet is to keep moving forward in the java course, you'll be opened up to a lot more concepts quickly. Once you're done with those I'd recommend you come back and look at this once you have a more broader concept of java, it will definitely take away the feeling like you're in a box feeling.
Thanks, I hope this helps.
reece
1,975 Pointsreece
1,975 PointsThanks man, I'll more forward and in the future try the method + return string way!