Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial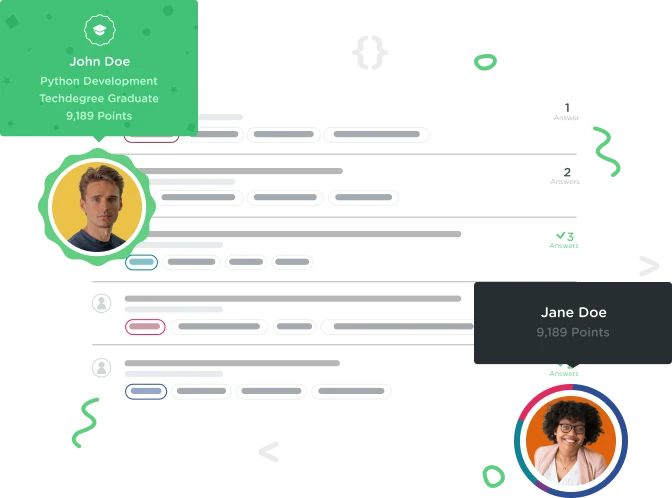

David Luces
397 PointsIs this too messy?
I don't have any problems reading it but then again I just wrote it. Are there any ways I can improve on this? A different POV would help.
//vars
var questions = [
[ 'What is the name of the program we are currently learning?', 'javascript'],
[ 'What is the name of the website we are using?', 'treehouse'],
[ 'What is 2+2?', 4]
];
var questionHTML;
var correctAnswers = 0;
var wrongAnswers = 0;
//print function
function print(message) {
document.write(message);
}
//question loop
for ( var i = 0; i < questions.length; i += 1) {
var questionsAnswer = prompt( questions[i][0] );
if( questionsAnswer.toLowerCase() === questions[i][1] || parseInt(questionsAnswer) === questions[i][1] ) {
alert('Correct!');
correctAnswers += 1;
} else {
alert('Wrong!');
wrongAnswers += 1;
}
}
questionHTML = 'You have answered ' + correctAnswers + ' questions correctly and ' + wrongAnswers + ' questions incorrectly!';
print(questionHTML);
Mod Note Added some forum markdown to format the code for Community Forum
2 Answers
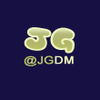
Jonathan Grieve
Treehouse Moderator 91,252 PointsThere's not much wrong with what you've done at all. You could maybe separate the longer concatenated strings into multiple lines in your text editor which might make the code a little easier to read but this is personal preference really.
One other thing you'll learn later on (not in this course) is template literals.... this is a relatively newer way to reference values and format strings in your code but as I say, that's for later.
As you get further into your coding journey you;'ll develop your own styles and preferences but good job on what you've done so far. :)
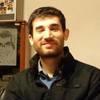
Axel Perossa
13,930 PointsIt looks perfectly fine to me, I did something similar but I made all the answers strings, and then checked simple equality in the if, not strict equality. I think your approach is better. Great job.
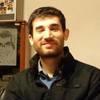
Axel Perossa
13,930 PointsI'm trying to comment the code also, at least short inline comments. It really helps in the long run, try it.
David Luces
397 PointsDavid Luces
397 PointsThank you for the feedback! I spaced out the long strings. It definitely makes it easier to read!