Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial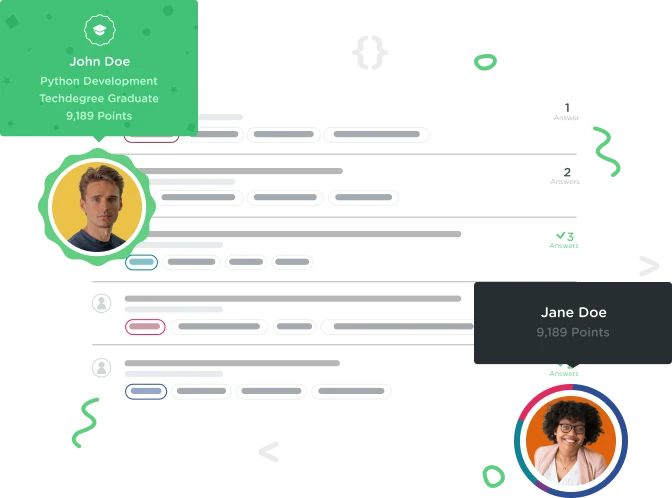
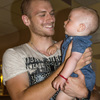
Lukas Vavro
7,211 PointsIs this way, correct?
function randomNumber(x, y) {
if (x < y) {
var firstNumber = y;
var secondNumber = x;
}
var vysledok = Math.floor(Math.random(x, y) * (6)) + 1;
return vysledok;
}
var firstNumber = prompt('Give me first number.');
var secondNumber = prompt('Give me second number.');
alert('Nahodne cislo medzi ' + firstNumber + ' a ' + secondNumber + ' je ' + randomNumber());
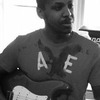
Samuel Webb
25,370 PointsWhy are you setting firstNumber and secondNumber to y and x in the randomNumber function? As a matter of fact that entire if statement serves no purpose.
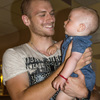
Lukas Vavro
7,211 PointsThanks for fixed code.
And If statement?
I want check this:
exemple User give firstNumber 2 and secondNumber 10.
so alert will be "Random number between 2 and 10 is ...." - So I think this is correct.
But if user give firstNumber 10 and secondNumber 2.
so alert will be "Random number between 10 and 2 is ...." - So I think this is wrong, when upper number is first. The "If statement" give lower number to firstNumber but I see that it does not work :/
Sorry for my english, I hope you understand. :/ :)
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsSo unfortunately your code won't work.
First, you can't pass any arguments to the Math.random()
function.
Second, it's a good idea to check which number is the higher one, but unfortunately you're not doing it correctly.
You would need to check which is greater, then use that particular parameter (either x
or y
) instead of the 6
in the formula for creating the random number that was in the Workspace to begin with: Math.floor(Math.random() * (6 - 1 + 1) + 1
and the lower number would be used instead of the first and last 1
in that formula (leave the + 1
within the parentheses).
So your function would look more like this:
function randomNumber(x, y) {
if (x < y) {
return Math.floor(Math.random() * (y - x + 1)) + x;
} else {
return Math.floor(Math.random() * (x -y + 1)) + y;
}
}
Third, you're trying to set variables both within and outside the function. As Dave mentioned in the video, these variables will have different scopes, and so they won't interact with each other. So the variables within the function never get changed from outside.
Fourth, as explained in a previous video, the prompt
function will always get the responses as strings, so you need to convert it to a number before you can use it in your randomNumber
function, by passing it into the parseInt
function:
var firstNumber = parseInt( prompt('Give me first number.' ) );
var secondNumber = parseInt( prompt('Give me second number.') );
Fifth, because your function expects two arguments to be passed to it (x
and y
), you need to pass those when you call it:
alert( randomNumber(2, 9) );
I'd suggest going back and revisiting past videos and challenges, maybe even do them a couple of times to make sure you fully understand it, as each stage builds on the last.
Happy coding! :)
Samuel Webb
25,370 PointsSamuel Webb
25,370 PointsI fixed your code so that it shows properly. Make sure you use the three back ticks before and after your code to make it show up like this.