Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial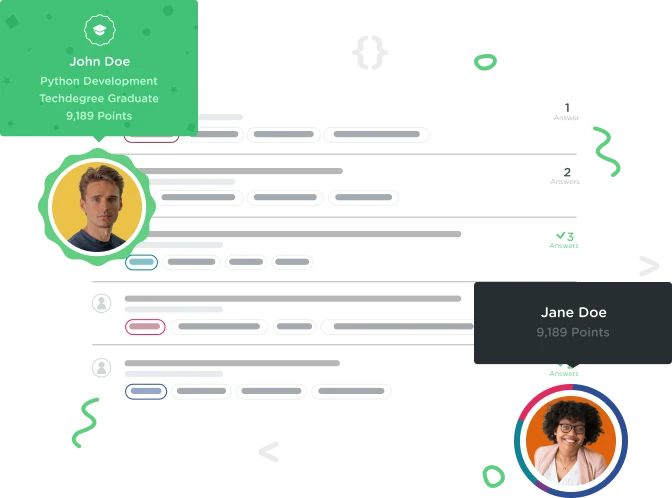
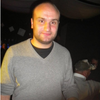
Vittorio Somaschini
33,371 PointsisDivisible function: Is this code good?
Hello guys.
Before looking at Amit Bijlani 's solution I produced this code for this exercise:
func isDivisible(num1: Int, num2: Int) -> Bool? {
let remainder = num1 % num2
if (remainder == 0) {
println("Divisible")
return true
} else {
println("Not Divisible")
return nil
}
}
isDivisible(5, 1)
I have now understood Amit's solution, but I would like to know if this code can be considered good too. Forget for a second that I am not really using the "true" and "nil" bool values, I am only returning them (just because the exercise asks for that). Would this piece of code be considered good?
I know it works but I haven't followed what we learned in these last videos precisely and I would like to have some opinions.
Thanks
Vittorio
7 Answers
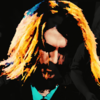
Justin Horner
Treehouse Guest TeacherHello Vittorio,
Your code is clean and explicit. I would say you certainly passed the challenge :)
Thanks for sharing. I hope this helps.

alexmalov
2,037 PointsI used a somewhat different solution.
func isDivisible (#firstNumber: Int, #secondNumber: Int) -> Bool? {
if firstNumber % secondNumber == 0 {
return true
} else {
return nil
}
}
if isDivisible(firstNumber: 2, secondNumber: 2) == true {
println("Divisible")
} else {
println("Not Divisible")
}

Daniel Mattingley
1,493 PointsHi Vittorio,
I've had a look through your code. Looks pretty straightforward, easy to understand and does the job efficiently and quickly. That's enough to judge it as good code! As you say, the return is unnecessary (and hence not great code!) but you address that and why you did it so no worries.
Good work!
Daniel

Simon Rood
1,096 PointsI came up with a different solution, but am wondering if this is as efficient as Vittorio's I now know the let result is unnecessary, I could just return true or false.
// Divisible or not?
func divideBy(#number1: Int, #number2: Int) -> Bool?{
let remainder = number1 % number2
if remainder == 0 {
let result = true
return result
} else {
let result = false
return result
}
}
if divideBy(number1: 8, number2: 3) == true {
println("Divisible")
} else {
println("Not Divisible")
}

Dimitrios Lotsaris
803 PointsBoth codes are clean and good. But, I guess, they are not what the teacher wants us to do. And both of them use more lines of code.

Phil Waligora
Courses Plus Student 3,083 PointsHere is my solution. I chose to check that we do not divide by zero. Using the ternary operator allows you to use fewer lines of code.
func isDivisible(#numerator:Int, #denominator:Int) -> Bool? {
if denominator != 0 {
return (numerator % denominator == 0) ? true : nil
}
return nil;
}
if let divisible = isDivisible(numerator: 100, denominator: 26) {
println("Divisible")
} else {
println("Not Divisible")
}

Balkaran Samra
1,341 PointsVittorio your solution is much more short and concise than mine and looks very good. Here is my code:
func isDivisible(#firstNumber: Int,#secondNumber: Int) -> Bool? {
if firstNumber % secondNumber == 0 {
return true
}
return nil
}
let firstNumberInput = 1300
let secondNumberInput = 100
if let result = isDivisible(firstNumber: firstNumberInput,
secondNumber: secondNumberInput) {
println("\(firstNumberInput) is divisible by \(secondNumberInput)")
} else {
println("\(firstNumberInput) is not divisible by \(secondNumberInput)")
}
EDIT: Just now finished off the video and it seems as if my code is similar to Amit's.