Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial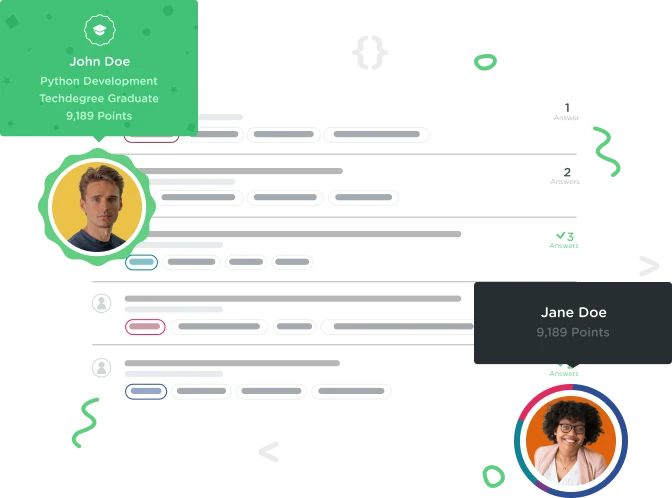
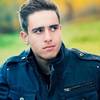
Unsubscribed User
14,547 PointsisNaN Check for Random Number Challenge, Part II
For the Random Number Challenge, Part II video, I came up with a bit different solution that seems to work.
In his solution, he uses:
if ( isNaN( lower ) || isNaN( upper ) ) {
}
my solution was to use:
if ( isNaN( lower || upper ) ) {
}
Is there a syntax or best-practice reason to rewrite the check twice? We're really just testing a bunch of different inputs to make sure they are numbers. I find my way reads clearer to me, and would be less writing with more parameters. I just wanted to make sure there wasn't anything wrong with my method.
1 Answer

Taylor Michel
15,634 PointsThere actually is a difference in the two. In your way you are using a shorthand statement where it will only return true if both values are NaN... The reason for this being that inside of your isNaN call you have 'lower || upper'. This is basically saying if 'lower' is NaN, null, or undefined use 'upper'. Thus if lower is NaN then it will pass 'upper' into isNaN() and if lower isn't NaN it will only pass 'lower' into isNaN().
i.e.
isNaN(24 || 24);
// isNaN = false ---> This will pass in the first 24 since it is valid, ignoring the second 24
isNaN(24 || NaN);
// isNaN = false ---> This will also pass in 24 since it is valid, ignoring the NaN
isNaN(NaN || 24);
// isNaN = false ---> This will see that NaN isn't valid and fallback on 24 passing that into isNaN, still resulting in false
isNaN(NaN || NaN);
// isNaN = true ---> This is the only case in which it will result in true because the first NaN fails and it falls back on the second one passing NaN into the isNaN function.
Hopefully this helps in understanding why the first way is the correct way of checking.
Taylor
Unsubscribed User
14,547 PointsUnsubscribed User
14,547 PointsThanks for the clarification. I'm still learning about the smaller details of how these functions work.
In your code you say isNaN(Nan || 24); evaluates to false, but in my code it seems to be evaluating to true. I just tested it separately. This would explain why I thought my code was working as I tried NaN || 24 in my code and my error message worked, but I didn't try 24 || NaN, in which case I would have seen it fail. It seems to really only matter if the left side is true. Maybe it can only be passed one argument?