Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial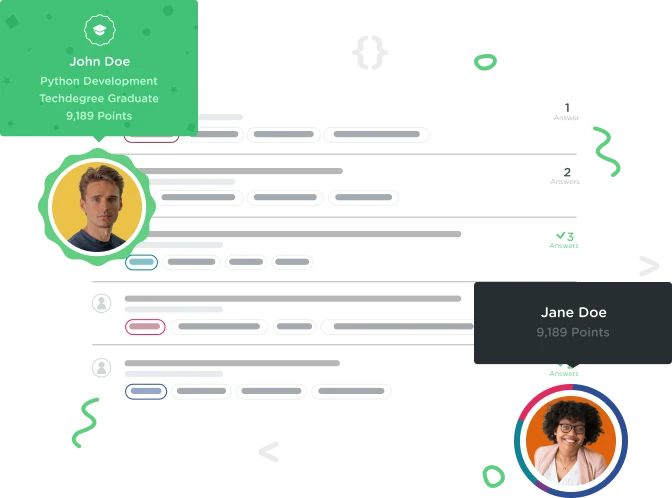

Bryan Smith
2,864 PointsisNaN error
function getRandomNumber( lower, upper ) {
if (isNaN(lower) || isNan(upper) ) {
throw new Error('Both arguments must be numbers.');
} else {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
}
console.log( getRandomNumber( 9, 24 ) );
console.log( getRandomNumber( 1, 100 ) );
console.log( getRandomNumber( 200, 'five hundred' ) );
console.log( getRandomNumber( 1000, 20000 ) );
console.log( getRandomNumber( 50, 100 ) );
the above code is what i entered.
I am getting this error message: Uncaught ReferenceError: isNan is not defined
2 Answers
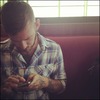
Erik McClintock
45,783 PointsBryan,
At a glance, it looks to me like this may be just a simple capitalization error. The error is telling you that you have tried using something that has not been previously defined; in this case, that something is 'isNan', and that is correct: 'isNan' has not been defined anywhere. You want to use 'isNaN', with the last 'n' capitalized. Try correcting that and see what you get.
Erik

Bryan Smith
2,864 Pointsomg thanks! haha. I was only looking at the first isNaN which was correct. debugging JavaScript can probably drive one crazy! looking for an extra space here, a mis-capitalized letter there.
Thanks!
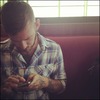
Erik McClintock
45,783 PointsOh, totally. Debugging ANY language can be a nightmare, due to how specific and exact we must be with our syntax everywhere. Once you've got something running that is hundreds or thousands of lines of code, it can absolutely be one of the worst things on the planet haha. Just always make sure to constantly save versions of your code and try to run/compile/refresh/whathaveyou to test every little change to every little thing (like, EVERY LITTLE CHANGE) as you're going along, and it will help to isolate the potential error zones in your code throughout production.
Happy coding!
Erik