Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial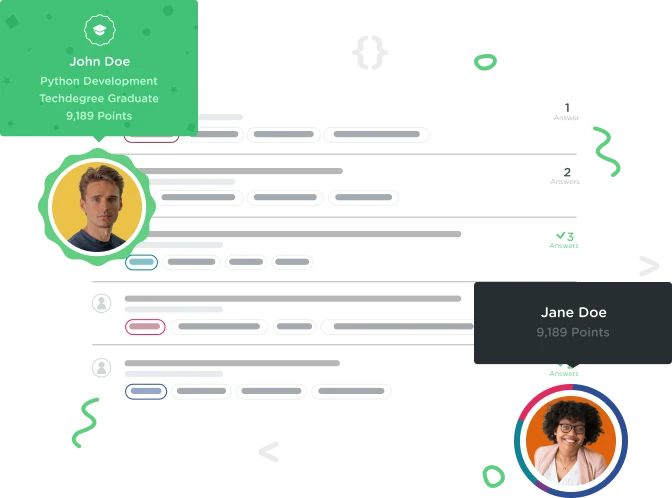
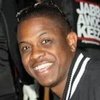
Carl Prude
Courses Plus Student 1,627 PointsIsn't == going to be more likely to equal a "true" value than ===? Bit confused on this test...
Had some issues understanding this... if the original code is saying they are not identical, why would === then make them identical? Isn't === more strict in evaluation than ==? My thought was that I should assign a value to myUndefinedVariable to = null. Then they would actually be equal. Just seemed a bit odd since we just discussed that === is more strict than ==...hope that makes sense!
6 Answers
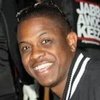
Carl Prude
Courses Plus Student 1,627 PointsEdit: Changed to best answer by Aaron Graham. This discussion contains a description of the actual problem.
Here is the challenge in its entirety:
Challenge task 1 of 1 The identical() method is being called but the variables are not identical. See if you can fix the code.
CODE:
<!DOCTYPE html> <html lang="en"> <head> <title> JavaScript Foundations: Variables</title> <style> html { background: #FAFAFA; font-family: sans-serif; } </style> <script src="ignore_this.js"></script> </head> <body> <h1>JavaScript Foundations</h1> <h2>Variables: Null and Undefined</h2>
<div id="container">
</div>
<script>
var myUndefinedVariable;
var myNullVariable = null;
if(myNullVariable == myUndefinedVariable) {
identical();
}
</script>
</body> </html>
ANSWER:
Add one more "=" to the following:
if(myNullVariable == myUndefinedVariable) { identical(); }
MY ISSUE: My issue is that in this SPECIFIC example, the ACTUAL variables are NOT identical (myNullVariable is null, and myUndefinedVariable is undefined) SO why would adding a "=" make this problem be correct? If the variables are not equal, and I evaluate with ===, shouldn't that result in a false? Since they actually aren't equal?
Unless we're saying that an undefined variable is equal to a null variable which doesn't sound right.

Aaron Graham
18,033 PointsYour understanding of the equality operators is correct, I think you are just misunderstanding the challenge. As it is, the identical() function will execute even if the values are not identical; null == undefined is true and the if statement executes the function. They want you to change the if statement so that the function only executes if the values are identical. Using a strict equal "===" does just that.

Robert Ho
Courses Plus Student 11,383 Points"They want you to change the if statement so that the function only executes if the values are identical."
So you are saying using "===" on "null" and "undefined" confirms that they are identical?

Aaron Graham
18,033 PointsRobert Ho - Just the opposite. inside the if statement, there is a function call:
if(myNullVariable == myUndefinedVariable) {
identical();
}
The challenge is saying that, with myNullVariable set to null, and myUndefinedVariable set to undefined, the identical() function is being executed. This is undesirable behavior. It should only execute if the values are identical. By changing the statement to:
if(myNullVariable === myUndefinedVariable) {
identical();
}
the identical() function only executes if myNullVariable and myUdefinedVariable are exactly equivalent, not just both falsy values. They don't want you to make the function execute, they want you to keep if from executing, since the two variables are not identical.

Robert Ho
Courses Plus Student 11,383 PointsAh i see! Thank you for clarifying that Aaron! I wish I could upvote your answer but you left a comment instead of a reply haha

Aaron Graham
18,033 PointsI can't remember what the original challenge had for variables, but the issue with using "==" instead of "===", is that there are many things that can be truthy. for example:
var myVar = null;
myVar == null; //true
myVar == undefined: //true
//But using strict equals
myVar === null: //true
myVar === undefined; //false
In other words, with "==" the values being compared are not necessarily identical. Hope that helps.
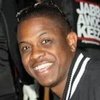
Carl Prude
Courses Plus Student 1,627 PointsHere is the challenge in its entirety:
Challenge task 1 of 1 The identical() method is being called but the variables are not identical. See if you can fix the code.
CODE: <!DOCTYPE html> JavaScript Foundations: Variables html { background: #FAFAFA; font-family: sans-serif; } JavaScript Foundations Variables: Null and Undefined
<div id="container"> </div>
<script>
var myUndefinedVariable;
var myNullVariable = null;
if(myNullVariable == myUndefinedVariable) {
identical();
}
</script>
ANSWER: Add one more "=" to the following:
if(myNullVariable == myUndefinedVariable) { identical(); }
MY ISSUE: My issue is that in this SPECIFIC example, the ACTUAL variables are NOT identical (myNullVariable is null, and myUndefinedVariable is undefined) SO why would adding a "=" make this problem be correct? If the variables are not equal, and I evaluate with ===, shouldn't that result in a false? Since they actually aren't equal?
Unless we're saying that an undefined variable is equal to a null variable which doesn't sound right.
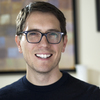
Timothy Hooker
15,323 PointsI think they really ought to rethink that quiz a little. It's confusing. I think it makes sense that you'd try to make the "if" statement execute. They should specify that the goal is for it not to execute because they are not identical. Or is this an encouragement to think critically?
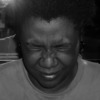
Tracy Mason
Courses Plus Student 2,446 PointsAgree. Leaving the question as "See if you can fix the code." brings up many possibilities. You can "fix the code" in several ways. Doesn't necessarily mean that it's what they are looking for. That annoyed me too.

April Daniels
2,435 PointsI agree. This quiz includes material that wasn't gone over in the lesson. I don't actually understand what he's asking. I try making both variables equal by defining them, but the only feedback I get is "bummer! null"
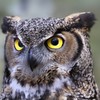
avremel
8,448 PointsI agree. I ran in to the exact same issue discussed here due to confusing instructions.
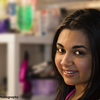
Zahscha Gonzalez
27,775 PointsThe reason why a === will make them identical is because they are both the same and have the same data type, that is very important to remember, the == will check if they are equal.
5 == "5" // true // This will return true because it converts the string into a number therefore they are equal.
5 === "5" //false //This is false because it is trying to identify if both values are identical (in type and value) but they are not.
Hope that helps.

Robert Ho
Courses Plus Student 11,383 PointsHm you've made a good point. I think this might be an error!
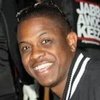
Carl Prude
Courses Plus Student 1,627 Points@Aaron Graham Thanks I see what you're saying. The challenge was to make the variables be identical in the formula, even though the example variables we're going to render a result of "false",
Robert Ho
Courses Plus Student 11,383 PointsRobert Ho
Courses Plus Student 11,383 PointsI think you are right about this and there is some sort of error. I don't think null should === undefined while you are using a stricter "===" vs "=="