Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial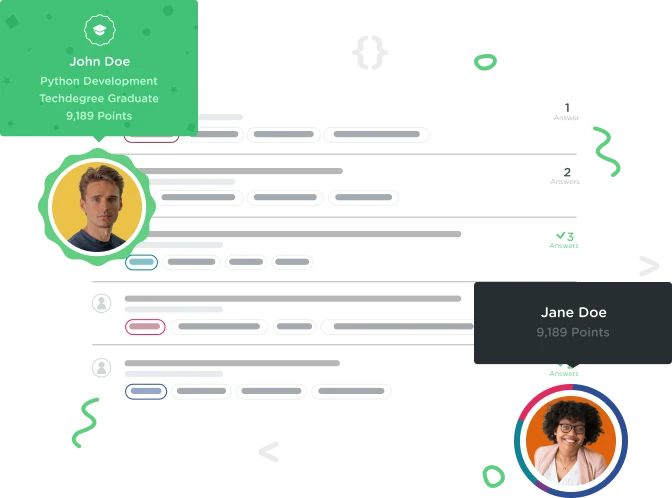

Ryan Jin
15,337 PointsIsn't NSArray immutable?
Isn't NSArray immutable, or unchangeable. If so, why in the video, Gabe could do something like
NSArray *movies = @["Multiple movies in this array..."];
movies = [movies deduped];
4 Answers

Ruggiero A
8,534 PointsYes NSArray are immutable. Keep in mind that a NSArray object can't change, alias its element can't change nor its dimension. So you can't do a thing like
[movies addObject:obj];
cause you need a NSMutableArray for that. But when you do
movies = [movies deduped];
you're not changing the object, you're changing the reference of the object that the variable movies keeps. Keep in mind that movies is not a NSArray itself, but a pointer to NSArray, and it's not a constant pointer. This means that the function deduped in movies, creates another NSArray and returns it, so when you're writing that line, you're creating and assigning to movies a new array.

Ruggiero A
8,534 PointsThe method provides a NSArray allocation and initialization. About the destruction, that's up to ARC (assuming it's enabled)

Ryan Jin
15,337 PointsSo if there is no that auto release pool thing in the code, there are basically two NSArrays when you call that deduped method?

Ruggiero A
8,534 PointsProbably the function should release the array after creating its copy.. I can't exactly answer here because I never programmed in Objective-C with no ARC (ARC is up since late 2010). Anyway most libraries use ARC, so it's best you use it in your projects. If a library doesn't use it, you can disable ARC for that library itself.

Ryan Jin
15,337 PointsSo the point is there are two NSArrays, is that right? And deleting the first one to conserve memory is being handled by the ARC?

Ruggiero A
8,534 PointsYes, the presence of two NSArrays though is very temporary, that's not gonna slow down your apps! ;D

Ruggiero A
8,534 PointsOops I just noticed I answered 2 more times instead of comment... Sorry I'm not used to this forum
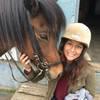
Naomi Schettini
1,608 PointsHow did the pointer know to point at NSArray movies??? That is where I am so confused in ObjC and I think it would help if I understood some of the mechanics behind the compiler..

Ruggiero A
8,534 PointsNSArray *movies
isn't a variable of type NSArray. Actually it is a variable pointer TO NSArray. In Objective-C there is no way to actually assign to a variable the value of an object (so NSArray movies
is just illegal, unlike C++).
For this way, the proper value of movies is not an array, or an object, but it is an address of memory.
When you call it methods, like [movies firstObject]
, you actually send a message to the object that movies
points to.
Objects are allocated in memory and variables or constants are just reference to them. (For this reason when no variable is pointing at an object in memory, it gets de-allocated by ARC)
Said this, the NSArray object allocated in memory, is IMMUTABLE. That means that you cannot modify its content, and above all you cannot modify its size.
What you can do, since a basic NSArray*
variable is just a variable pointer (just as a basic int*
variable), it can change value-address (the value of a pointer is an address).
When you do movies = [movies deduped];
, the deduped function is creating another object for you, which not depends on movies itself, but is a modified copy of it. After the assignment, movies points to a different object, while the old object which movies used to point to, gets de-allocated by ARC. If ARC was disabled, the disallocation should have happened in deduped function.
Ryan Jin
15,337 PointsRyan Jin
15,337 PointsSo does that mean the pointer is mutable, but the NSArray is immutable? If so, doesn't this take up more memory while a pointer is meant to use less memory?
Ruggiero A
8,534 PointsRuggiero A
8,534 PointsYeah the pointer is mutable, it would be different if you were declaring it as static const. It doesn't take up more memory cause a pointer keeps a simple 4-bytes or 8-bytes value (a RAM address), it does not change much if it's mutable or not. NSMutableArrays tend to use more memory because the size of elements is variable, so the allocation is not optimized and they tend to use more memory than they would need.
Ryan Jin
15,337 PointsRyan Jin
15,337 PointsDoes it that dedupe method delete the original NSArray and create a new one? If not, doesn't that make it very inefficient?