Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial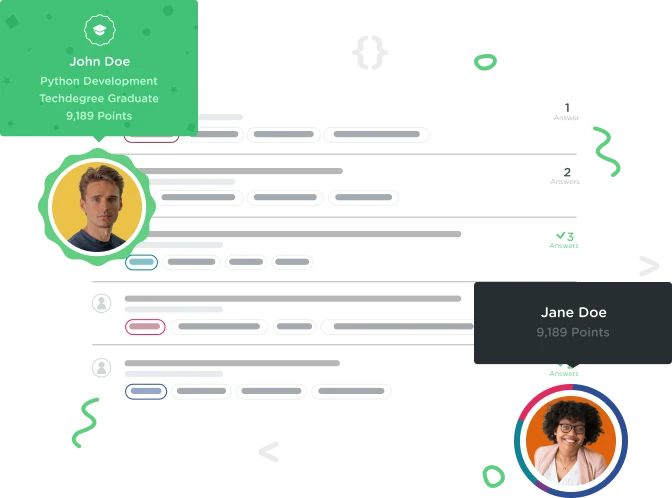

Ruthba Yasmin
3,558 PointsisSolved method
Game.java
public class Game{
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer){
mAnswer = answer;
mHits = "";
mMisses = "";
}
private char validateGuess(char letter) {
if (! Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0){
throw new IllegalArgumentException(letter + " has already been guessed");
}
return letter;
}
public boolean applyGuess(String letters) {
if(letters.length() == 0) {
throw new IllegalArgumentException("No letter found");
}
if(letters.length() >= 2){
throw new IllegalArgumentException("Please enter one letter at a time to guess your word");
}
//char firstLetter = letters.charAt(0);
//return applyGuess(firstLetter);
return applyGuess(letters.charAt(0));
}
public boolean applyGuess(char letter) {
letter = validateGuess(letter);
boolean isHit = mAnswer.indexOf(letter) >= 0;
if(isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter : mAnswer.toCharArray()) {
char display = '_';
if(mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
public int getRemainingTries() {
return MAX_MISSES - mMisses.length();
}
public boolean isSolved() {
//We are using a chaining method here: we already have a method that's keeping track of our current progress, which getCurrentProgress and it returns a String (---- if it's not completed and letters that have already hit will be revealed). we use this method and apply chaining method, i.e calling another method on the result returned by this method. -1 means check to see if there are no dashes in the returned string anymore..
return getCurrentProgress().indexOf('-') == -1;
}
public String getAnswer() {
return mAnswer;
}
}
Prompter.java
import java.io.Console;
public class Prompter{
private Game mGame;
public Prompter(Game game){
mGame = game;
}
public void play() {
while (mGame.getRemainingTries() > 0 && !mGame.isSolved()) {
displayProgress();
promptForGuess();
}
gameResult();
}
public void gameResult(){
if (mGame.isSolved()) {
System.out.printf("Congratulations you won with %d tries remaining!\n",
mGame.getRemainingTries());
} else {
System.out.printf("Bummer the word was %s. :(\n", mGame.getAnswer());
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
//char guess = guessAsString.charAt(0);
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
System.out.printf("%s. Please try again.\n",iae.getMessage());
}
}
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s\n", mGame.getRemainingTries(), mGame.getCurrentProgress());
}
}
Hangman.java
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
prompter.play();
}
}
it never enters the while loop in the Prompter.java play() method (and I'm suspecting isSolved is returning true therefore !isSolved)
As soon as I run the program the console simply prints:
Congratulations you won with 7 tries remaining!
I don't know where I'm going wrong. Can't completely debug the program but I tried my best. Seeking help!
2 Answers

Ruthba Yasmin
3,558 PointsThanks a lot Logan, but I figured it out the exact problem!
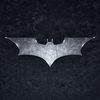
Logan R
22,989 PointsI'm not able to really debug your Java code at the moment, but it looks like to me, your problem is getCurrentProgress()
method. We can tell because in gameSolved, it checks mGame.isSolved()
, and it's returning true.
What I would try is printing out result
in getCurrentProgress()
right before the return.
My guess is (without being able to run the program or modify the code) that you have this statement, return getCurrentProgress().indexOf('-') == -1;
, which is checking to see if any '-' are in the word, but you are adding in '_
' for missing letters, not '-', which would return true, because there are no '_
'.
Hope this helps!