Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial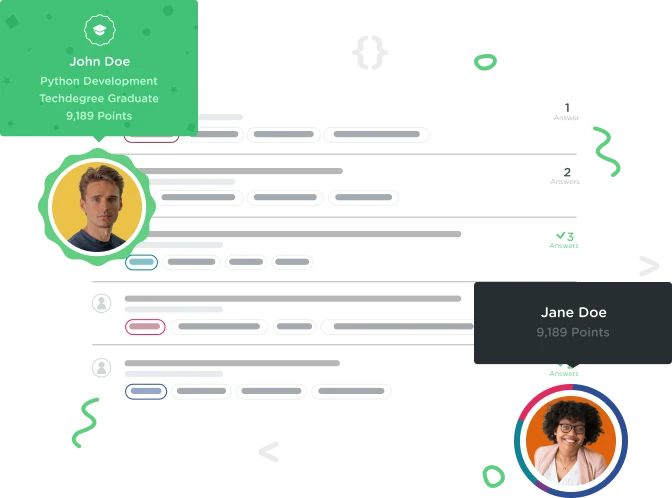

Unsubscribed User
Full Stack JavaScript Techdegree Student 1,198 PointsIssue calculating the # of correct answers
/*
- Store correct answers
- When quiz begins, no answers are correct */ let correctAnswers = 0;
// 2. Store the rank of a player let playerRank;
// 3. Select the <main> HTML element let results = document.querySelector('main');
/*
- Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers */ const question1 = prompt('What is the biggest country in the world?'); const question2 = prompt('How many continents are in the world?'); const question3 = prompt('Where is the Amazon river located?'); const question4 = prompt('Which country has more pandas?'); const question5 = prompt('What is the highest mountain');
const answer1 = 'russia'; const answer2 = '6'; const answer3 = 'brazil'; const answer4 = 'china'; const answer5 = 'mount everest';
if (question1 === answer1) { correctAnswers += 1; } else if (question2 === answer2) { correctAnswers++; } else if (question3 === answer3) { correctAnswers += 1; } else if (question4 === answer4) { correctAnswers += 1; } else if (question5 === answer5) { correctAnswers += 1; } else { correctAnswers = 0; }
/*
- Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown */
if (correctAnswers === 5) { playerRank = 'Gold'; } else if (correctAnswers === 3 && correctAnswers === 4) { playerRank = 'Silver'; } else if (correctAnswers === 1 && correctAnswers < 3) { playerRank = 'Bronze'; } else { playerRank = 'No crown'; }
// 6. Output results to the <main> element
results.innerHTML = <h1>You got ${correctAnswers} out of 5 questions correct. Crown earned: ${playerRank}</h1>
;
for some reason when I run the code, it only shows 1 out of questions correct as an output, any idea why? I checked the syntax and the variable and I can't find problem.
Thank you
5 Answers
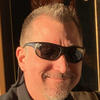
Peter Vann
36,427 PointsHi Ismael!
You were on the right track!?!
I fixed several things, to make it work, though...
You had some key code commented out.
You didn't need all the else statements.
Your innerHTML string, being a template literal, needed to be surrounded by backticks.
This should work:
/*
// 1. Store correct answers
When quiz begins, no answers are correct */
let correctAnswers = 0;
// 2. Store the rank of a player (declare the variable, really)
let playerRank;
// 3. Select the <main> HTML element
let results = document.querySelector('main');
/*
Ask at least 5 questions
Store each answer in a variable
Keep track of the number of correct answers */
const question1 = prompt('What is the biggest country in the world?');
const question2 = prompt('How many continents are in the world?');
const question3 = prompt('Where is the Amazon river located?');
const question4 = prompt('Which country has more pandas?');
const question5 = prompt('What is the highest mountain');
const answer1 = 'russia';
const answer2 = '6';
const answer3 = 'brazil';
const answer4 = 'china';
const answer5 = 'mount everest';
if (question1 === answer1) { correctAnswers += 1; }
if (question2 === answer2) { correctAnswers++; }
if (question3 === answer3) { correctAnswers += 1; }
if (question4 === answer4) { correctAnswers += 1; }
if (question5 === answer5) { correctAnswers += 1; }
/*
Rank player based on number of correct answers
5 correct = Gold
3-4 correct = Silver
1-2 correct = Bronze
0 correct = No crown */
if (correctAnswers === 5) { playerRank = 'Gold'; } else if (correctAnswers === 3 && correctAnswers === 4) { playerRank = 'Silver'; } else if (correctAnswers === 1 && correctAnswers < 3) { playerRank = 'Bronze'; } else { playerRank = 'No crown'; }
// 6. Output results to the <main> element
results.innerHTML = `<h1>You got ${correctAnswers} out of 5 questions correct. Crown earned: ${playerRank}</h1>`;
I hope that helps.
Stay safe and happy coding!
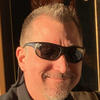
Peter Vann
36,427 PointsYes, (that was the biggest issue) a logic error (which trips me up often, so don't feel bad!?!).
You want to test every answer, so no else statements are needed.
BTW, I just noticed that you could have used correctAnswers++; for all five cases, but correctAnswers += 1; is virtually identical (just slightly more typing!?! LOL)
One tip: to debug something like this, I put console.log statements all over the code to test/see the variable values as they are set, and often it will point out the problem.
Oh and I often test JS code here, just to see it in action, I did in this case:
https://www.w3schools.com/js/tryit.asp?filename=tryjs_array
Copy this code:
<!DOCTYPE html>
<html>
<body>
<main>
</main>
<script>
/*
Store correct answers
When quiz begins, no answers are correct */
let correctAnswers = 0;
// 2. Store the rank of a player
let playerRank;
// 3. Select the <main> HTML element
let results = document.querySelector('main');
/*
Ask at least 5 questions
Store each answer in a variable
Keep track of the number of correct answers */
const question1 = prompt('What is the biggest country in the world?');
const question2 = prompt('How many continents are in the world?');
const question3 = prompt('Where is the Amazon river located?');
const question4 = prompt('Which country has more pandas?');
const question5 = prompt('What is the highest mountain');
const answer1 = 'russia';
const answer2 = '6';
const answer3 = 'brazil';
const answer4 = 'china';
const answer5 = 'mount everest';
if (question1 === answer1) { correctAnswers += 1; }
if (question2 === answer2) { correctAnswers++; }
if (question3 === answer3) { correctAnswers += 1; }
if (question4 === answer4) { correctAnswers += 1; }
if (question5 === answer5) { correctAnswers += 1; }
/*
Rank player based on number of correct answers
5 correct = Gold
3-4 correct = Silver
1-2 correct = Bronze
0 correct = No crown */
if (correctAnswers === 5) { playerRank = 'Gold'; } else if (correctAnswers === 3 && correctAnswers === 4) { playerRank = 'Silver'; } else if (correctAnswers === 1 && correctAnswers < 3) { playerRank = 'Bronze'; } else { playerRank = 'No crown'; }
// 6. Output results to the <main> element
results.innerHTML = `<h1>You got ${correctAnswers} out of 5 questions correct. Crown earned: ${playerRank}</h1>`;
</script>
</body>
</html>
Paste it in the left pane of that link (overwrite what's there), run it, and you'll see it in action!?!
Again, I hope that helps.
Stay safe and happy coding!

Unsubscribed User
Full Stack JavaScript Techdegree Student 1,198 PointsGot ya!, thank you so much for the tips, appreciate it :)

seema chavesta
12,174 Points/*
- Store correct answers
- When quiz begins, no answers are correct */ let correctAnswer = 0;
// 2. Store the rank of a player let playerRank;
let answer = prompt('Is HTML programming language? yes or no'); if(answer === 'no'){ correctAnswer = 1; playerRank = "Bronze"; } answer - prompt('is javaScript is programming language? yes or no'); if(answer === 'yes'){ correctAnswer = 2; playerRank = "Silver"; }
answer = prompt('is Css is programming language? yes or no'); if(answer === "no"){ correctAnswer = 3; playerRank = "Silver"; }
answer = prompt('is React programming language? yes or no'); if(answer === 'No'){ correctAnswer = 4; playerRank = "Silver"; }
answer = prompt('is Css stand for casecading style sheet? yes or no'); if(answer === 'yes'){ correctAnswer = 5; playerRank = "Gold"; }
let main = document.querySelector('main');
if(correctAnswer === 1){
main.innerHTML = <h1> You have answered ${correctAnswer} out of 5 and you have earned ${playerRank} </h1>
;
}else if(correctAnswer === 2){
main.innerHTML = <h1>You have answered ${correctAnswer} out of 5 and you have earned ${playerRank} </h1>
;
}else if (correctAnswer === 3 || correctAnswer === 4){
main.innerHTML = <h1>You have answered ${correctAnswer} out of 5 and you have earned ${playerRank} </h1>
;
}else if(correctAnswer === 5){
main.innerHTML = <h1> You have answered ${correctAnswer} out of 5 and you have earned ${playerRank} </h1>
;
}else {
main.innerHTML = <h1> You have earned no crown </h1>
;
}

Unsubscribed User
Full Stack JavaScript Techdegree Student 1,198 PointsGreat, now I understand, so basically else if clause was preventing the whole code block to run right?