Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial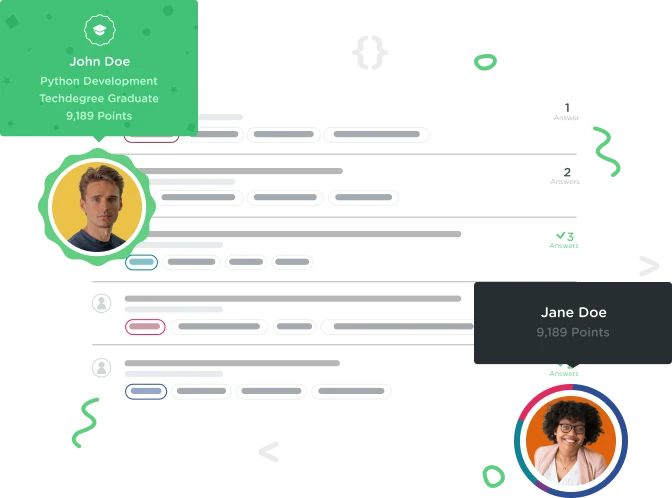
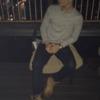
Aleksey Titov
Courses Plus Student 530 PointsIssue spotted.
For some reason when ever I put something other then "dork" I still get a message telling me not to do so.
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
String howOld = console.readLine("How old are you: ");
int age = Integer.parseInt(howOld);
if (age < 13) {
//Insert exit code
console.printf("Sorry you must be atleast 13 years of age to run this program\n");
System.exit(0);
}
String name = console.readLine("What is your name? ");
String lastName = console.readLine("Also what is your last name? ");
String friendName = console.readLine("What is your friends name? ");
if (friendName.equals("dork")); {
console.printf("Sorry but you cannot name your friend dork\n\n");
System.exit(0);
}
2 Answers
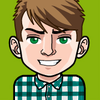
Harry James
14,780 PointsHey Aleksey!
The issue is being caused by the semicolon at the end of the if statement here:
if (friendName.equals("dork")); // <--- Here!
We don't need to end the if statements with a semicolon, so you can go ahead and remove it then the challenge should pass.
Let me know if you run into any more issues :)
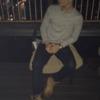
Aleksey Titov
Courses Plus Student 530 PointsWow, you guys reply really fast.
It is now working, thankyou. Just one more question, how come we dont need to end it with a semicolon? and is it for all if statements?
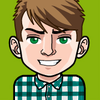
Harry James
14,780 PointsHey again Aleksey!
Thanks a bunch :)
The reason we don't need a semicolon is because in Java, it would be classed as an empty statement if we were to use a semicolon here. Basically, that means that the code does nothing! The semicolon is used by the compiler to signify the end of a statement, so if you end the if statement immidiately, to the compiler, that just looks like an empty if statement. Here's what it looks like to the compiler:
if (friendName.equals("dork")) {
; // End of statement, we're done here.
}
{
console.printf("Sorry but you cannot name your friend dork\n\n");
System.exit(0);
}
Notice how the code then carries on? That's why you still got the message showing "Sorry but you cannot name your friend dork", even though you could have entered any value.
A good way to know when and when not use the semicolon here is that when you are using curly braces { }
, you don't need to use a semicolon. Here's another example:
while(true) {
console.printf("Example");
}
This is a while loop, and you only need the semicolons on each statement inside the curly braces.
Hopefully this should explain how this works for you but if there's still something you don't understand, feel free to give me a shout! :)
You can also tag me on these forums with @Harry James
which notifies me and I'll make sure to write an answer to your question if somebody else hasn't beat me to it :)
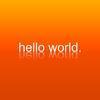
Tomasz Sporys
11,258 Pointsyes, all if statements. That's just the syntax
Aleksey Titov
Courses Plus Student 530 PointsAleksey Titov
Courses Plus Student 530 PointsFull source, sorry.