Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial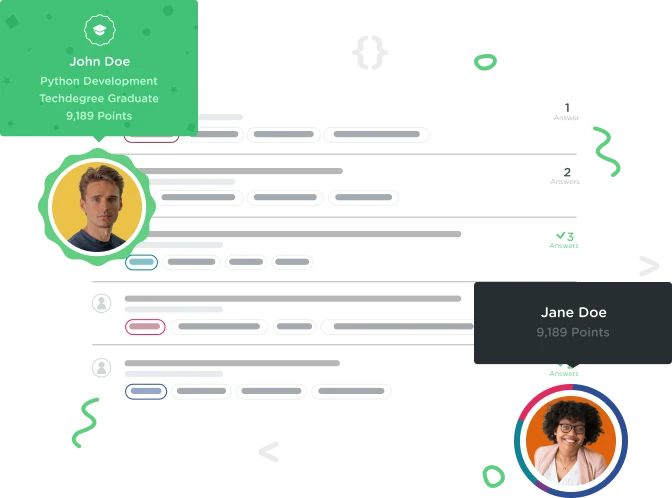

Joanna Deirmendjian
674 PointsIssue using the getColor method using System.out.printf to get color of object
This is the code:
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
new GoKart("Red");
System.out.printf("The color is %s\n",
getColor());
}
}
This is the error:
./Example.java:7: error: cannot find symbol getColor()); ^ symbol: method getColor() location: class Example 1 error
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
new GoKart("Red");
System.out.printf("The color is %s\n",
getColor());
}
}
3 Answers
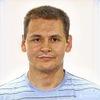
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsAs your compiler suggests:
./Example.java:7: error: cannot find symbol getColor()); ^ symbol: method getColor() location: class Example 1 error
When you write in code, getColor()
compiler is trying to find method getColor
in the class where the code executed.
Obviously, there is no getColor()
method in the Example
class.
Hint: you should run this method on the class, where this method exists ...

Joanna Deirmendjian
674 PointsSo mColor? I thought the private code on GoKart class would mean I use getColor? Anyway. Totally confused now.

Joanna Deirmendjian
674 PointsWhen I initially did this code in the previous exercise:
new GoKart("Red");
The challenge stated I was correct. I guess that is where my error lies.
Joanna Deirmendjian
674 PointsJoanna Deirmendjian
674 PointsHmmm.. Yes, totally lost.
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsAlexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsWhen you create
new GoKart
object you have to save it to re-use later:Now because we want to get the color of particular "kart" that we created and not some other kart, we have to use
getColor()
method on the "kart" we createdIf you feel confused, please re-watch video before. In it Craig is doing exactly the same: