Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial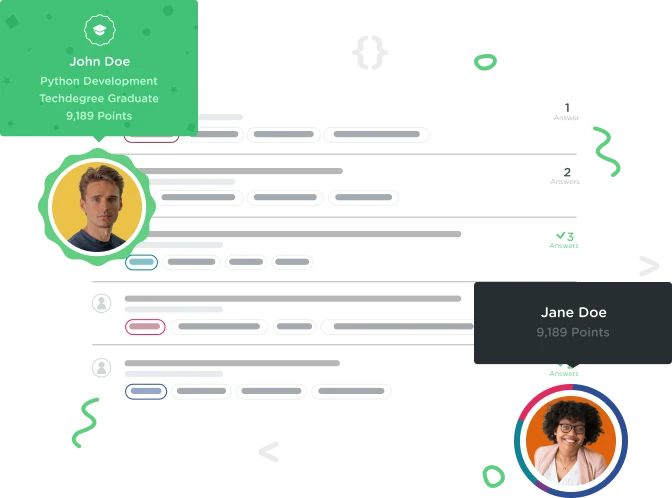
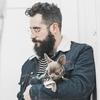
Alexander D
8,685 PointsIssue with button. Promise is not definied.
Hello there,
I am trying to work on a personal project, but following the instructions of the video. The structure of the code you're about to see is very similar to what Guil is explaining. At the bottom of my code, there's a button that should fire the request send(), and show possible errors with .then and .catch methods.
However, because I am enclosing these methods inside the button, it seems Javascript is not recognizing the promise anymore.
Here is my CodePen:
https://codepen.io/DeMichieli/pen/oNvpOqG
Here is my code:
//button
let btn = document.getElementById("btn")
//wrapper off all. We're doing this into a function
function send() {
//this is the wrapper for the promise and we define the promises
const promise = new Promise((resolve, reject) => {
//we start creating the XML request and we open the document
const xhr = new XMLHttpRequest();
xhr.open("GET", 'https://api.icndb.com/jokes/random');
//2 conditons - onload and onerror
xhr.onload = () => {
if (xhr.status === 200 && xhr.readyState === 4) {
let jokes = JSON.parse(xhr.responseText);
let arr = jokes.value; //this is very important as converts the JSON into an object
let toWrite = arr.joke
document.getElementById('text').innerHTML = toWrite;
//it's necessary to state resolve to receive information about the success
resolve(xhr.response);
} else {
reject(Error(xhr.statusText)); // status is not 200 OK, so reject
}
};
xhr.onerror = () => {
reject(Error('Error fetching data.'))
}
xhr.send();
});
//-- function send() closing --
}
btn.addEventListener("click", (event) =>{
send();
promise.then((result) => {
console.log('Got data!', result);
}).catch((error) => {
console.log('Error occurred!', error);
});
});
if the promise.then((result) etc is included before the closing bracket of the send() function, all works fine. However, when this is included inside an event handler it doesn't log the issue anymore.
In the video Guil explains (minute 4:00) he's adding these instructions inside a button. Here's the video:
https://teamtreehouse.com/library/from-callbacks-to-promises
Thanks!
2 Answers
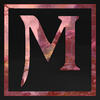
Matthew Lang
13,483 PointsYour promise
variable is within the local scope of the send
function so you can not access it outside of it (in an event handler for example).
You should try returning the promise in the send
function and then use the send
function to define your promise. Here's a brief example.
function send() {
return new Promise((resolve, reject) => {
/* your promise code here */
})
}
btn.addEventListener("click", event => {
let promise = send() // we return Promise in send(), so let's store it here
promise.then(result => { // we can now use it here :)
console.log('Got data!', result);
}).catch(error => {
console.log('Error occurred!', error);
})
})
It is worth noting that this may not be how Guil intended to achieve this however this is how I would.
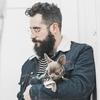
Alexander D
8,685 PointsMatthew Lang Thanks for taking the time to look at my code, it works perfectly!
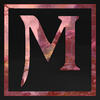
Matthew Lang
13,483 PointsNo problem. :)