Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial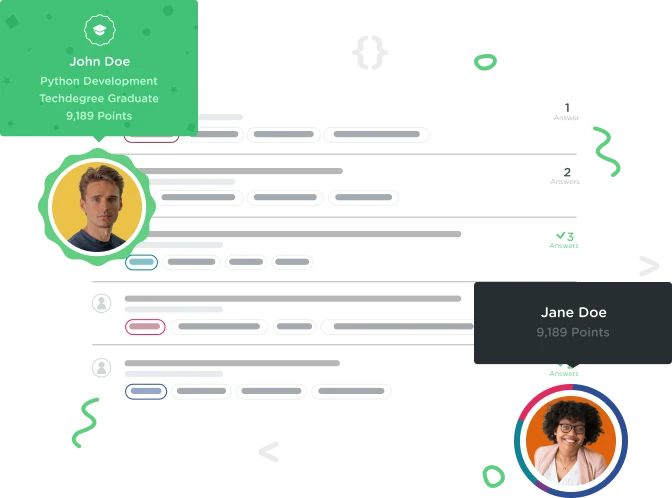
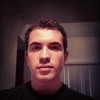
Samuel Stephen
8,493 PointsIssue with my Random Number Generator
// Make a program that accepts two values and generates a random number from the first to the second
// 1. prompt dialogue to collect user input
var user1num = prompt("Choose a number");
// 2. parselnt function to convert the input to an integer
document.write(parseInt(user1num) + ", ");
// 1. prompt dialogue to collect user input greater than the first number entered
var user2num = prompt("Choose a number greater than your first number.");
// 2. parselnt function to convert the input to an integer
document.write(parseInt(user2num));
// 3. Math.random method to create a random number
var dieroll = Math.round(Math.random() * parseInt(user2num) + parseInt(user1num));
alert("You rolled a " + dieroll);
The code works if you stick to what the program prompts you for, but if your first input is greater than the second input, problems happen. I want the program to return a number between 10 and 3, but it won't happen.
If anyone can help I would be so happy.
2 Answers
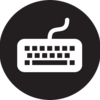
Schweine FEin
2,671 PointsYou could check first which one is the bigger with an if statement and than put it in an other variable. like so:
// UserInputOne and User InputTwo have already been set
if ( UserInputOne > UserInputTwo ) {
var small = UserInputTwo;
var big = UserInputOne;
} else {
var big= UserInputTwo;
var small = UserInputOne;
}
var dieroll = Math.round(Math.random() * small + big);
Well thats just a concept idea not really working code. But something like this could solve your problem.
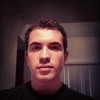
Samuel Stephen
8,493 Points// Make a program that accepts two values and generates a random number from the first to the second
// 1. prompt dialogue to collect user input
var user1num = prompt("Choose a number");
// 2. parselnt function to convert the input to an integer
document.write(parseInt(user1num) + ", ");
// repeat steps 1 & 2
var user2num = prompt("Choose another number.");
document.write(parseInt(user2num));
/* 3. Using Conditional statements, organize the input values
so the generated number is between the input values. */
if ( user1num > user2num ) {
var small = user2num;
var big = user1num;
} else {
var small = user1num;
var big = user2num;
};
// 4. Math.random method to create a random number
var dieroll = Math.round(Math.random() * parseInt(big)) + parseInt(small);
alert("You rolled a " + dieroll);
Thanks to Luca Fuhl, my program works using the Conditional statements!
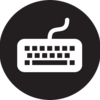
Schweine FEin
2,671 Pointscongrats for getting the solution of your problem :)
Samuel Stephen
8,493 PointsSamuel Stephen
8,493 PointsThanks so much, I'm going to try what you said!