Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial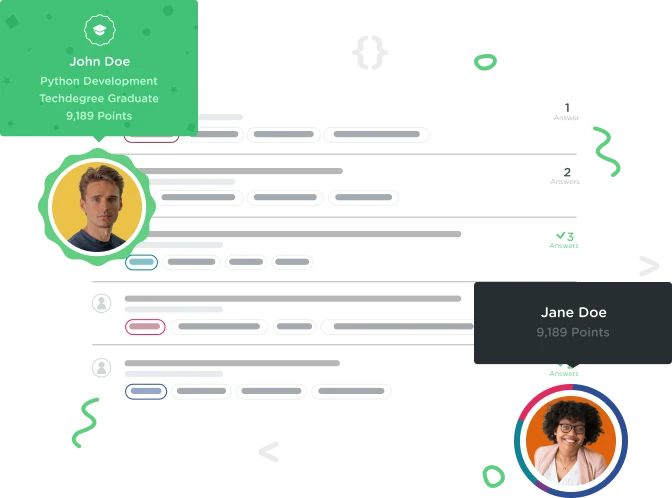
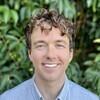
Asher Orr
Python Development Techdegree Graduate 9,408 PointsIssue with Python Code Challenge: a variable is not being recognized by the interpreter (getting a NameError.)
Hi everyone! I'm on the Technical Interview Prep section in the Python Techdegree.
Here's the challenge:
"Take a look at the example data structure below. Create a function called breeds. It will receive 1 parameter, a data structure like the one below. Return a list of all the pet breeds a person has. For example, using the data structure below, the function should return ['American Shorthair', 'Pitbull']."
In the interpreter, this is what you see:
# {
# 'name': 'Javier Hernandez',
# 'pets': [
# {
# 'name': 'Kitty',
# 'breed': 'American Shorthair'
# },
# {
# 'name': 'Buzz',
# 'breed': 'Pitbull'
# }
# ],
# 'classes': ('Math', 'Science', 'Art')
# }
# enter your code below
Since this dictionary is commented out and not assigned to a variable, I was puzzled by how to access it with a function.
I decided to remove the pound symbols and assign it a name, the_dict.
Here's my code:
# {
# 'name': 'Javier Hernandez',
# 'pets': [
# {
# 'name': 'Kitty',
# 'breed': 'American Shorthair'
# },
# {
# 'name': 'Buzz',
# 'breed': 'Pitbull'
# }
# ],
# 'classes': ('Math', 'Science', 'Art')
# }
# enter your code below
the_dict = {
'name': 'Javier Hernandez',
'pets': [
{
'name': 'Kitty',
'breed': 'American Shorthair'
},
{
'name': 'Buzz',
'breed': 'Pitbull'
}
],
'classes': ('Math', 'Science', 'Art')
}
def breeds(data_structure):
pet_breeds = []
for entry in the_dict["pets"]:
pet_breeds.append(entry["breed"])
return pet_breeds
breeds(the_dict)
When I run this code in Visual Studio Code, it works. The function returns ['American Shorthair', 'Pitbull']
But when I click "Check Work" on the Code Challenge, I get this:
Traceback (most recent call last): File "", line 55, in test_results File "/workdir/utils/challenge.py", line 24, in execute_source exec(src) File "", line 38, in File "", line 34, in breeds NameError: name 'the_dict' is not defined
I'm confused as to why the interpreter thinks the_dict is not defined. Am I answering this question in a way that is confusing the code challenge's automated grader/checker?
Any insights would be appreciated. Thank you all for your time!
1 Answer
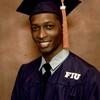
Dane Parchment
Treehouse Moderator 11,075 PointsTLDR; Solution is
def breeds(data_structure):
pet_breeds = []
for entry in data_structure["pets"]:
pet_breeds.append(entry["breed"])
return pet_breeds
Read further to understand why
So the real reason you are running into this issue is due to how you aren't using your arguments. The reason the code works for you is because you have a dictionary defined in the outer scope called the_dict
. However, the point of the challenge is to write a function that can handle that sort of input, not that you have to create the input itself, if you understand what I mean.
Basically the function is supposed to stand on it's own like so:
def breeds(data_structure):
pet_breeds = []
for entry in the_dict["pets"]:
pet_breeds.append(entry["breed"])
return pet_breeds
With that in mind the reason your challenge is failing is because you are relying on a variable defined outside of the function scope. Which wouldn't be used in testing the function because they'll be supplying their own data to it, not using your specific dictionary.
To fix this you just need to use arguments to be a placeholder for that data. You already did this with the data_structure
argument, but didn't actually use it, instead electing to use the dictionary you created outside of it.
To fix this, just use the argument.
def breeds(data_structure):
pet_breeds = []
for entry in data_structure["pets"]:
pet_breeds.append(entry["breed"])
return pet_breeds
Asher Orr
Python Development Techdegree Graduate 9,408 PointsAsher Orr
Python Development Techdegree Graduate 9,408 PointsWhat a fantastic answer! That was well explained, and I learned a lot from reading your post.
Thank you, Dane!