Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial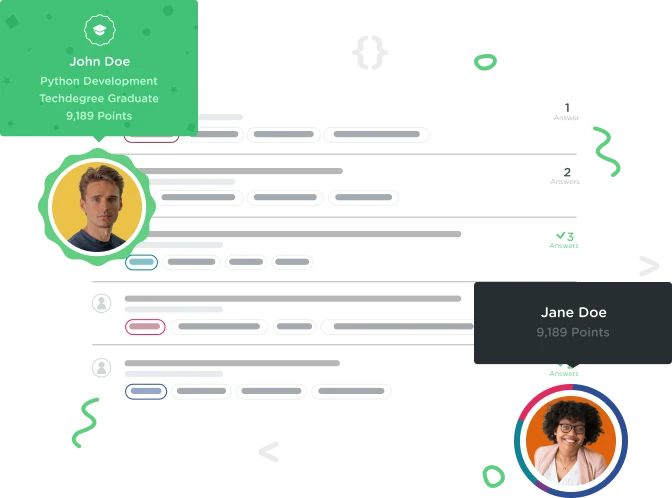

Jegnesh Gehlot
5,452 PointsIssue with requesting the video thumbnail using MPMoviePlayer
I am trying to parse the JSON data which has video urls and the title. After parsing the data I am showing the video thumbnail in a UITableviewCell. The problem is that when the data is parsed and when its time to request for the thumbnail the NSNotification selector method is never been called. Here is the code:
override func viewDidLoad() { super.viewDidLoad() getJson_results() }
override func viewWillDisappear(animated: Bool) {
NSNotificationCenter.defaultCenter().removeObserver(self)
}
func videoThumbnailIsAvailable(notification: NSNotification) {
println("Flag1") //the control never reaches here
var thumbnail = notification.userInfo?[MPMoviePlayerThumbnailImageKey] as UIImage
self.temp_image = thumbnail
}
func videodurationIsAvailable(notification: NSNotification) {
var val = moviePlayer?.duration
self.temp_duration = val!
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int { return length_array; }
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 1
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("Cell", forIndexPath: indexPath) as WPR_Main
cell.imgView?.image = get_Media_thumbnail(NSURL(string:self.url[indexPath.row])!)
cell.titleLabel?.text = self.titles[indexPath.row]
return cell
}
func get_Media_thumbnail(url: NSURL) -> UIImage {
moviePlayer? = MPMoviePlayerController(contentURL: url)
NSNotificationCenter.defaultCenter().addObserver(self,
selector: "videoThumbnailIsAvailable:",
name: MPMoviePlayerThumbnailImageRequestDidFinishNotification,
object: nil)
moviePlayer?.requestThumbnailImagesAtTimes([1.0], timeOption:.NearestKeyFrame )
return self.temp_image
}
1 Answer

Jegnesh Gehlot
5,452 PointsThe best way to achieve this is to use AVAssetImageGenerator instead of using requestThumbnailImagesAtTimes. AVAsset also allow to download the images asynchronously which is quite faster and applicable to the requirement. Here is the code:
var urlAsset = AVURLAsset(URL: NSURL(string:self.url[indexPath.row])!, options: nil)
if(urlAsset.tracksWithMediaType(AVMediaTypeVideo).count > 0){
var imageGenerator = AVAssetImageGenerator(asset: urlAsset)
imageGenerator.appliesPreferredTrackTransform=true
let durationSeconds = CMTimeGetSeconds(urlAsset.duration)
let midPoint = CMTimeMakeWithSeconds(durationSeconds/2, 1)
var error:NSError? = nil
var actualTime = CMTimeMake(0, 0)
imageGenerator.generateCGImagesAsynchronouslyForTimes( [ NSValue(CMTime:midPoint) ], completionHandler: {
(requestTime, thumbnail, actualTime, result, error) -> Void in
if result == AVAssetImageGeneratorResult.Succeeded {
dispatch_async(dispatch_get_main_queue()) {
var thumbnailImage = UIImage(CGImage: thumbnail)
cell.imgView?.image = thumbnailImage
}//dispatch
}//if
else {
var thumbnailImage = UIImage(named: "video-backup.png")
cell.imageView?.contentMode = .ScaleAspectFit
cell.imgView?.image = thumbnailImage
}
})