Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial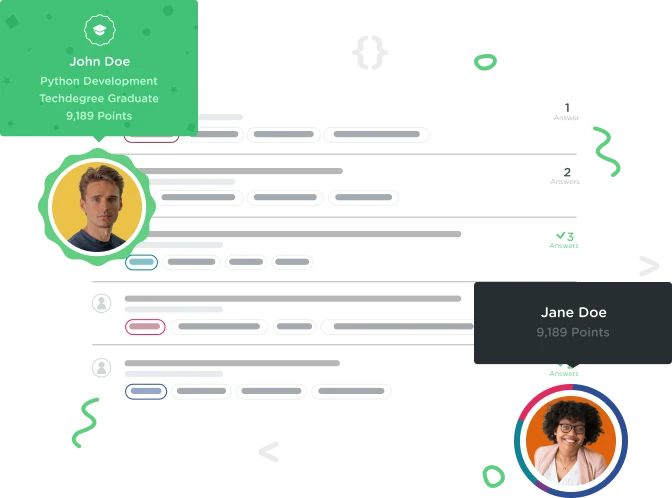

John Locken
16,540 PointsIssue with score_yatzy at end of Object Oriented Python challenge
Trying to figure out why my code won't work, Treehouse keeps telling me it's getting the wrong score for my yatzy hand. The question is:
The instructions are: Great! Let's make one more scoring method! Create a score_yatzy method. If there are five dice with the same value, return 50. Otherwise, return 0.
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(self, hand):
return sum(hand)
def score_yatzy(self, hand):
compare = int(hand[0])
for item in hand:
if int(item) == compare:
continue
else:
return 0
return 50
1 Answer

John Locken
16,540 PointsI answered my own question! I told it to break after return 0, not sure if it's necessary. I also moved return 50 a row closer to home. Here is how that ended up looking:
def score_yatzy(self, hand):
compare = int(hand[0])
for item in hand:
if int(item) == compare:
continue
else:
return 0
break
return 50
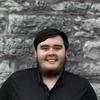
Michael Hulet
47,913 PointsGreat job! Just to provide some explanation to this, but the break
statement isn't necessary. In fact, it will never be run. This is because the return
right before it instantly ends the functions execution and gives back 0
as the result, and no code after it will run. In fact, that's what was wrong with your code before. return 50
was indented to be inside the loop, so when the loop ran, it would always return 50
on the very first iteration, and it would never even check any of the other numbers. Now that you've moved that line to be outside the loop, it'll check each number, return 0
if there's a mismatch, and then return 50
if they're all the same and it got to the end of the loop without return
ing 0
Kyan Shlipak
5,986 PointsKyan Shlipak
5,986 PointsNevermind, I have been having a super annoying bug where it takes like 20 tries until the same code passes. It finally worked with no edits.