Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial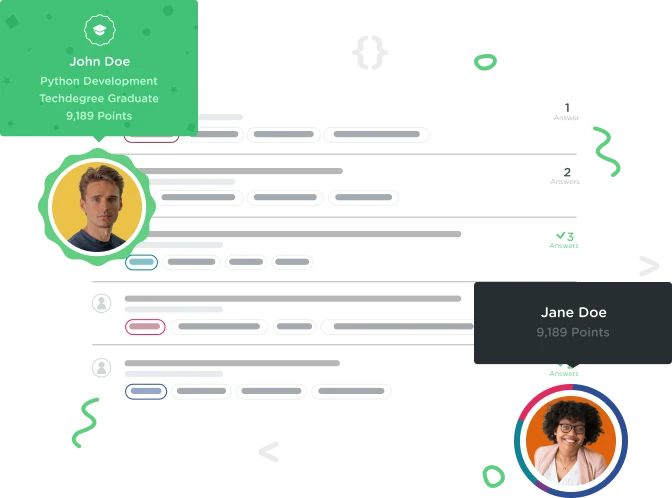
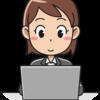
ilovecode
8,071 PointsIssue with the float returning 1 or 2 on tens
I am creating a calculator for when I insert a float number that it calculates and converts to currency in the Harry Potter world (you know Galleons, Sickles and Knuts).
1 Galleon has 17 Sickles. 1 Sickle has 29 Knuts.
When I enter the floating number the function calculates how many galleons and sickles and knuts there are. But if it is a number like 12.20 for example, the result must be 12 sickles and 20 knuts. But instead I get 12 sickles and 2 knuts. The same happens for 10 as well, it returns 1 instead of 10. There will never be 30 as a floating point, so it is only for 10 and 20.
My code seems very bad and full of 'if statements', so if you can suggest any better practices and improvements, go ahead, I will appreciate it.
Thanks in advance.
function priceSeparated (price) {
let parts = price.toString().split('.');
let galleons = 0;
let sickles = 0;
let knuts = 0;
if (parts[0] >= 17) {
galleons = Math.floor(parts[0]/17);
sickles += parts[0] % 17;
}
if(parts[1] >= 29) {
knuts = Math.floor(parts[1]/29);
knuts += parts[1] % 29;
}
if (parts[0] < 17) {
galleons = 0;
sickles += parts[0] % 17;
}
if (parts[1] <= 28) {
knuts = Math.floor(parts[1]/29);
knuts += parts[1] % 29;
console.log(knuts);
}
if (galleons === 0) {
console.log(`${sickles} sickles and ${knuts} knuts`);
return `${sickles} sickles and ${knuts} knuts`;
}
else if (sickles === 0) {
console.log(`${galleons} galleon/s and ${knuts} knuts`);
return `${galleons} galleon/s and ${knuts} knuts`;
}
else if (knuts === 0) {
console.log(`${galleons} galleon/s and ${sickles} sickles`);
return `${galleons} galleon/s and ${sickles} sickles`;
}
else {
console.log(`${galleons} galleon/s, ${sickles} sickles and ${knuts} knuts`);
return `${galleons} galleon/s, ${sickles} sickles and ${knuts} knuts`;
}
}
priceSeparated(12.20);
//returns 12 sickles and 2 knuts
1 Answer
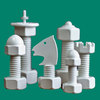
Steven Parker
231,007 PointsThe normal string representation of the floating-point value 12.20 is "12.2". To get the "20" you need to use a different function to create a string with a fixed precision:
let parts = price.toFixed(2).split('.');
And you can generalize the formula to eliminate the conditionals:
let galleons = Math.floor(parts[0]/17);
let sickles = parts[0] % 17 + Math.floor(parts[1]/29);
let knuts = parts[1] % 29;
ilovecode
8,071 Pointsilovecode
8,071 PointsGreat, thanks!