Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial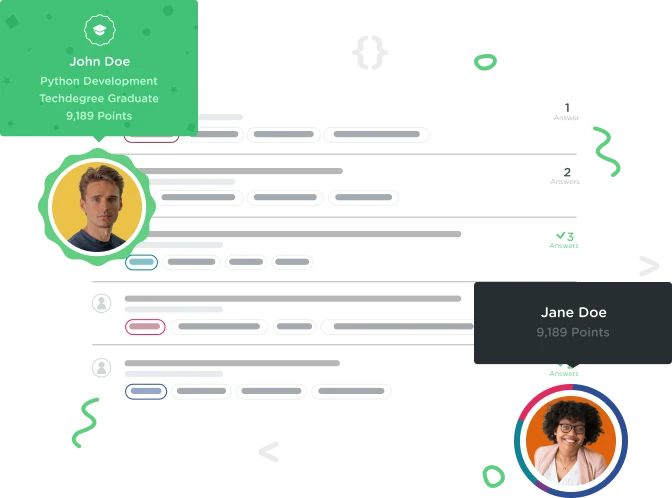
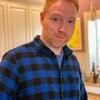
Jason Arnold
22,110 PointsIssues with { 0p }
Having some issues with importing the 'Op' operators
When using the code from the lesson I get an error that Op is undefined
When I use the code from the Sequelize docs I get an error of s.replace is undefined
Here is the code I have at the beginning of app.js
const db = require('./db');
const { Movie, Person } = db.models;
//const { Op } = require('sequelize');
const { Op } = db.Sequelize;
I don't have both the third and fourth line running at the same time, I just put them both in for this example. I'm running version 5.22.4 of Sequelize. Any ideas? Thanks.
1 Answer
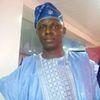
Adebayo Ojo
23,661 PointsCheck the content of your index.js file if it follows the below:
const Sequelize = require('sequelize');
const sequelize = new Sequelize({
dialect: 'sqlite',
storage: 'movies.db',
logging: false
});
const db = {
sequelize,
Sequelize,
models: {},
};
db.models.Movie = require('./models/movies.js')(sequelize);
db.models.Person = require('./models/person.js')(sequelize);
module.exports = db;
JASON LEE
17,351 PointsJASON LEE
17,351 PointsHi Jason.
Doing some debugging and I had a similar issue where it was not recognizing
Op
(not yet in use). In the debugger console I set a watch onOp
and it was showing up correctly, until after it ran the asynchronous queries such asconst movies = await Movie.findAll({ ....
. Afterwards,Op
changed to "Uncaught ReferenceError ReferenceError: Op is not defined".Now, when I use
Op
in the async query section, Op works fine.Here is my code at the top
Now here is without using
Op
Now here is with using
Op
Just bizarre.