Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial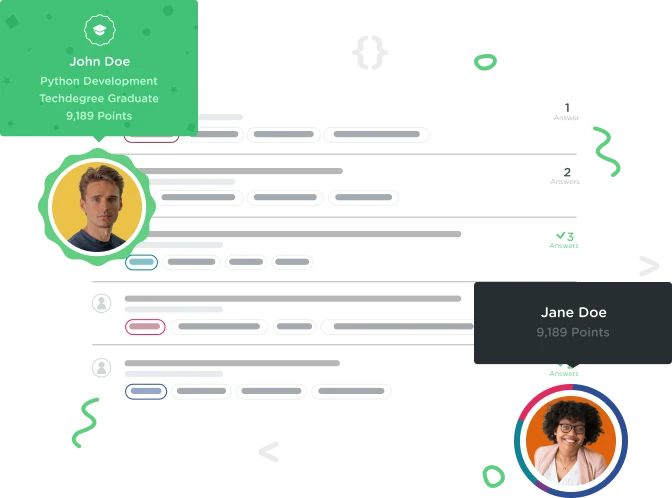

Jon Mendelson
10,897 Pointsissues with code challenge: introducing conditional statements (javascript)
I eventually found the solution that the site wanted me to use, though it is not as good (and considering that we're taught the solution in the lesson before it, my solution should work). However, I ran into issues on the javascript conditional statements challenge.
I had:
var answer = prompt("What is the best programming language?");
if ( answer.toLowerCase() === "javascript")
{
alert("You are correct.");
}
This told me it broke part 1, so I went back to part 1 and attempted to run the code. It gave me the following error: TypeError: 'null' is not an object (evaluating 'answer.toLowerCase')
After doing some research, I updated the code to:
var answer = prompt("What is the best programming language?");
if (answer !== null && answer.toLowerCase() === "javascript")
{
alert("You are correct.");
}
This made it compile for part one, but when I attempted to compile it for part 2 i got the error "Did you use === to compare the answer with the string 'JavaScript' like this: answer===='JavaScript'"
The code ran fine aside from the error message and did what it was supposed to, but I was being told I was wrong.
Figured I'd point out the bug.
4 Answers
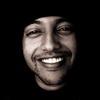
miikis
44,957 PointsHey Jon,
The answer should actually look like this:
var answer = prompt('What is the best programming language?');
if( answer === 'JavaScript' ) {
alert("You are correct");
} else {
alert("JavaScript is the best language!");
}
The Code Challenge just wanted you to say 'JavaScript' and not 'javascript'. Clever of you to find a way around it though.
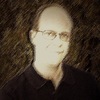
Jason Anders
Treehouse Moderator 145,858 PointsI think the challenges are designed to be very specific and highly picky to help us learn to pay attention to even the smallest detail. While this example may not be practical in real-life applications, it forces us to carefully read the instructions, and then carefully enter the code to achieve that specific result. Attention to detail is very important in coding, and in any kind of design really, so these challenges are just helping us gain the necessary skill set we will need in the real world.
Keep Coding! :)
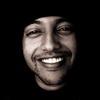
miikis
44,957 PointsThe subtle difference between a bug and a feature hehe I agree with you though Jason
Pedro Sousa
Python Development Techdegree Student 18,546 Pointswell.... I see your point...
But the challenge is actually telling you to check if the answer is exactly "JavaScript". So, i just assumed to literally try the answer variable against "JavaScript" (with the capital S) and it passed.
Pedro Sousa
Python Development Techdegree Student 18,546 PointsHello Jon,
I think I had the same "bug" as you a few days back on this challenge. It took me a while.
We need to always remember that the string comparison in Javascript is case sensitive. So, "j" is different than "J".
That should solve the bug ;)

Jon Mendelson
10,897 Pointsright, I found the solution, but my solution took that into account with the toLowerCase(), and accounted for if the user input null (hit escape). I was merely pointing out that since we were taught to use the toLowerCase() function right beforehand to resolve this very problem, it should be considered a correct answer.
Jon Mendelson
10,897 PointsJon Mendelson
10,897 Pointsright, I did eventually find that solution, it's just inferior to the solution that we were taught in the lesson beforehand (what if the person responding doesn't use a capital 's'?). I was just pointing out that there is a bug that a valid solution is marked as incorrect.
miikis
44,957 Pointsmiikis
44,957 PointsOh yeah, I get what you're saying. This type of thing happens a lot in the Code Challenges. I think it's because its just too tedious (and unnecessary) to hardcode every possible technically-correct answer into the Challenge. Often you're asked a very specific thing and if you deviate from that in the smallest of ways, it won't pass. It's not that bad though; you kinda get used to it after a while.