Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial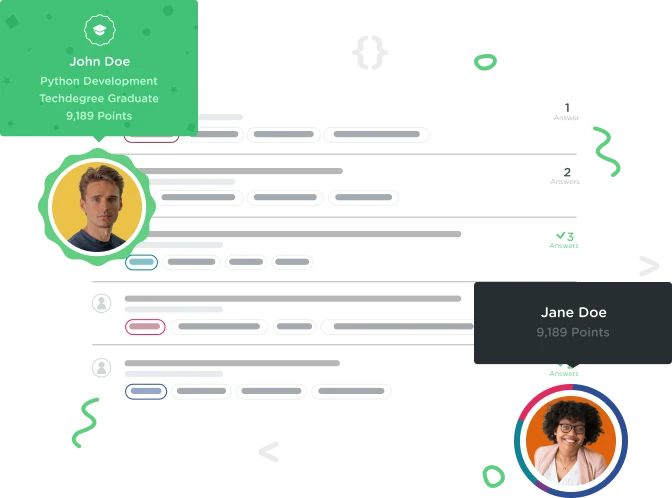
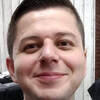
Sergio Andrés Herrera Velásquez
Courses Plus Student 23,765 PointsIssues with my morse_code challenge, I added some comments pseudocode for the thought process I am following, any idea?
def morse_code(word):
morse_dict = {
'a': 'dot-dash',
'b': 'dash-dot-dot-dot',
'c': 'dash-dot-dash-dot',
'd': 'dash-dot-dot',
'e': 'dot',
'f': 'dot-dot-dash-dot',
'g': 'dash-dash-dot',
'h': 'dot-dot-dot-dot',
'i': 'dot-dot',
'j': 'dot-dash-dash-dash',
'k': 'dash-dot-dash',
'l': 'dot-dash-dot-dot',
'm': 'dash-dash',
'n': 'dash-dot',
'o': 'dash-dash-dash',
'p': 'dot-dash-dash-dot',
'q': 'dash-dash-dot-dash',
'r': 'dot-dash-dot',
's': 'dot-dot-dot',
't': 'dash',
'u': 'dot-dot-dash',
'v': 'dot-dot-dot-dash',
'w': 'dot-dash-dash',
'y': 'dash-dot-dash-dash',
'z': 'dash-dash-dot-dot'
}
#lowercase the word to match letters in the dict
word=word.lower()
#create empty list to store letters
letters=[]
#loop through the letters in word
for letter in word:
#replacing every letter in the word
encoded_word=letter.replace(morse_dict.value)
#appending the letter to the list
letters.append(encoded_word)
#returning the list as a join string
return letters.join('-')
# enter your code below
2 Answers

Caleb Kemp
12,755 PointsYou have a lot of good things going on here and you are most of the way there. Take away, a lot of the problem is syntax errors.
I was able to solve the problem, and could just post the solutions, however, I know you want the practice of looking at something that doesn't work, and figuring out how to fix it. So, I'll just give a few (hopefully helpful) suggestions.
1 . If you hit the 'show preview' button on the challenge, you will see you are getting the following error
AttributeError: 'dict' object has no attribute 'value'
This is because to get the value from dict, it should be written as morse_dict[input], not morse_dict.value
2 . The replace method requires 2 inputs (original, replacementValue), not just one like written.
3 . Your .join statement was written backward. You currently have list.join(string), it needs to be written in the form (string).join(list)
4 . In python, indentations matter a whole lot, how your return statement is currently placed, it is returned after just the first letter. Think about how it could be placed so that it won't return until the whole loop has finished.
There you have it, fixing 2 lines of code is all that separates you from a working solution :)
Additional thoughts.
I'm not sure why we need encoded_word=letter.replace(morse_dict.value)
, couldn't we just skip that and have letters.append(morse_dict[input])
directly? Just a thought, hope it helps.
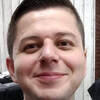
Sergio Andrés Herrera Velásquez
Courses Plus Student 23,765 PointsHi Caleb Kemp, thank you for the suggestions, I finally passed the task, here is the code that passed with the changes needed and to the encoded_word part, I usually track the changes through variables, so that I can also test the code as I go( figuring out where the ball dropped), but you are right.I removed it and applied the replace as appropiate, appended the letter to the lettes and moved the return statement one space to match the for, that way, it returned the final word.
Again, Thank you.
lowercase the word to match letters in the dict
word=word.lower()
#create empty list to store letters
letters=[]
#loop through the letters in word
for letter in word:
#replacing every letter in the word
letter.replace(letter, morse_dict[letter])
#appending the letter to the list
letters.append(morse_dict[letter])
#returning the list as a join string
return ('-').join(letters)

Caleb Kemp
12,755 PointsThat makes sense, I also think part of the reason why you found it difficult is that the challenge didn't let you use "print" statements (I found that annoying at least ). Great job sticking with it and getting it solved.