Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial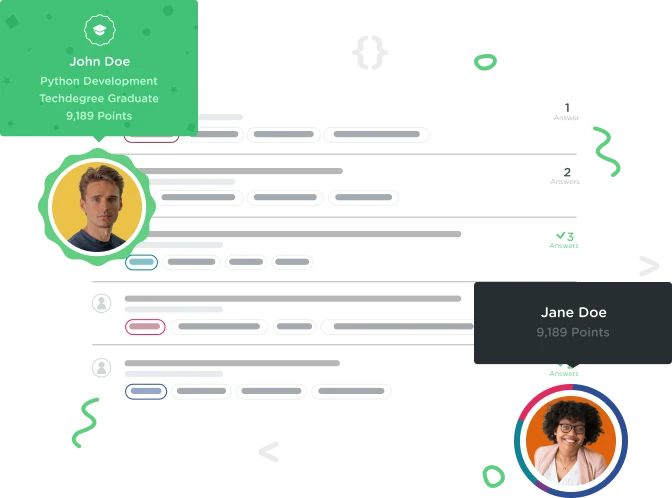

Timothy Villaraza
394 PointsIt almost seems like we should ALWAYS be using the function "try" while writing code just so that we can handle errors.
I hope I'm able to explain my question clearly enough,
Like the title suggests, my impression of the "try" function is that we should ALWAYS begin our code with it (of course with keeping style in mind). Almost like it could be used as a work around for a lot of our errors instead of rewriting our lines of code to fix them.
Is it even good practice to use them? I have no idea how regularly we should use them and if there are situations where they shouldn't be bothered with at all.
My knowledge in python or any other language is limited to this point of the track, so try to keep it in layman terms if possible!
4 Answers

Richard Li
9,751 PointsActually, in my understanding, a lot of the build-in function and methods already have traceback and try for you to use. That's why they are giving you the error feedback on what's wrong.
I mainly use Try_Except in handling any user input and give them feedback.
NEVER TRUST ANY USER'S INPUT

Jasper Peijer
45,009 PointsAs Richard said never trust user input. People will try to make the application crash in any way. Most other code would be better to not have a try except because YOU are the one using that code and it would be better to know what went wrong when you make a mistake. Once your code is free of mistakes there's no try except needed except ofcourse where there's user input.
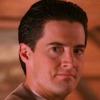
akoniti
1,410 PointsI think it depends on the specific input you're expecting as well. For example, I've been writing a text-based dungeon crawler, and the user chooses which door to go through next by inputting the color. Since there are only a very limited number of inputs that makes sense, I handle it like this by defining those explicitly. Here's the function for Room 5 in the dungeon:
def room5func():
player_visited [4] = 1
global last_room
global inventory
last_room = 5
print ("\n[You are in Room 5]\nYou see a blue door and a purple door.\n")
direction = input("What would you like to do?\n")
if 'blue' in direction or 'Blue' in direction or 'BLUE' in direction:
room2func()
elif 'purple' in direction or 'Purple' in direction or 'PURPLE' in direction:
room6func()
elif ('map' in direction or 'Map' in direction or 'MAP' in direction) and inventory[0] == 1:
map_func()
else:
print("Please try again\n")
room5func()
I'm sure I'll soon learn more efficient ways to do pretty much everything above, I'm very new to programming, but this example shows that it's not always specific Python errors that may be an issue, it could also be that the user inputs something that is simply out of context for the input request.

Tilak Muruduru Divakar
6,459 PointsYou can use this to compare strings by ignoring the case. 'blue' in direction.lower()
.lower() will throw an AttributeError if direction has a NoneType object.
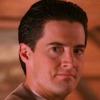
akoniti
1,410 PointsThanks Tilak! The .lower method is super helpful in the context of the ignoring case in user input!
Tilak Muruduru Divakar
6,459 PointsTilak Muruduru Divakar
6,459 PointsWe can also use it if we want to catch the traceback error that a built-in function throws and print a meaningful message to the user.