Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial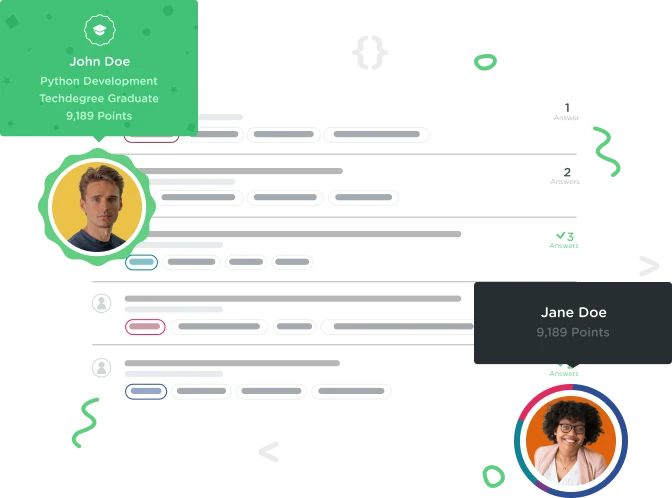
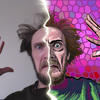
Seth Johnson
15,199 PointsIt appears to be working not *quite* like it should...
So after having been walked through it, everything is making sense to me and I think is working like it should, with one exception. Admittedly it's one where I zig and Guil zags, but I thought I'd investigate.
Here's my JS:
const myListTitle = document.querySelector('h1');
const desc = document.querySelector('.desc');
const myListItems = document.querySelector('ul');
const newItem = document.createElement('li');
const inputElements = document.querySelectorAll('input');
const deleteButton = document.createElement('button');
const extraContent = document.querySelector('.extra');
const container = document.querySelector('.container');
// 1: Set the text of the <h1> element
myListTitle.textContent = "Stuff that which I have achieved only a barely adequate Skill level";
// 2: Set the color of the <h1> to a different color
myListTitle.style.color = "tomato";
// 3: Set the content of the '.desc' paragraph
// The content should include at least one HTML tag
desc.innerHTML = "A list of things of which I <em>should</em> have a greater skill level";
// 4: Set the class of the <ul> to 'list'
myListItems.className = 'list';
// 5: Create a new list item and add it to the <ul>
newItem.innerHTML = "<input> Playing Smash Bros.";
myListItems.appendChild(newItem);
// 6: Change all <input> elements from text fields to checkboxes
for (let i = 0; i < inputElements.length; i += 1) {
inputElements[i].type = 'checkbox';
}
// 7: Create a <button> element, and set its text to 'Delete'
// Add the <button> inside the '.extra' <div>
deleteButton.textContent = "Delete";
extraContent.appendChild(deleteButton);
// 8: Remove the '.extra' <div> element from the DOM when a user clicks the 'Delete' button
deleteButton.addEventListener('click', () => {
container.removeChild(extraContent);
});
The index.html is exactly the same as Guil's. The main difference is that for my const inputElements
, I used the .querySelectorAll('input')
method, and it does work for turning the elements into checkboxes except for the newItem
input, which I have a feeling is what's causing the issue. I don't have any errors show up in my console (I'm using safari). Any thoughts?
1 Answer
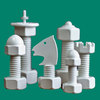
Steven Parker
229,732 PointsThe difference in using "getElementsByTagName" or "querySelectorAll" to select the elements is in the kind of object returned, but what also matters is when the selection is performed.
In the video code the selection is made after the new element has been added, so it will be included. But in the code above, the selection is made at the beginning, so the new item is not included. So moving the selection to where it is done in the video would fix the issue.
Using the other method, even without moving it, would also fix the issue because "getElementsByTagName" returns a live HTMLCollection, so it would update itself when the new item was added.
Willemijn B
5,577 PointsWillemijn B
5,577 PointsI used the same approach in declaring all variables at the top (as we're instructed after all :-)), and fixed this problem by using
let
instead ofconst
when declaring, then re-defining it in assignment 6. Came here to ask if there is another solution to this problem. Would usinggetElementsByTagName
be the preferred approach over the way I did it?Steven Parker
229,732 PointsSteven Parker
229,732 PointsYou didn't show your code, but just in general any selector function that gets you what you need is OK.