Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial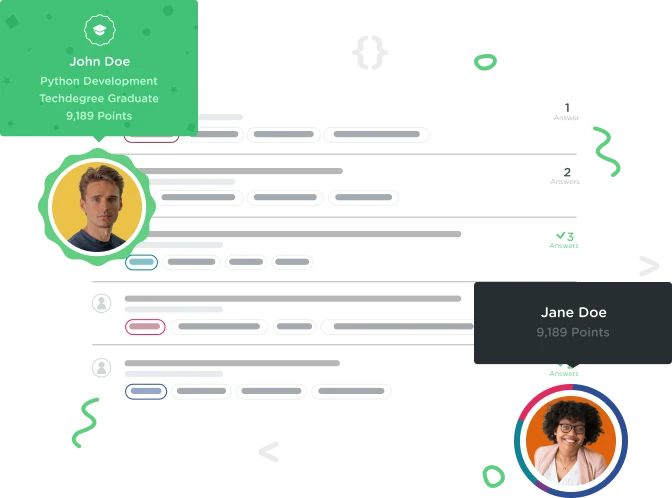

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 Pointsit did not work for me, why?
const usernameInput = document.getElementById("username"); const passwordInput = document.getElementById("password"); const telephoneInput = document.getElementById("telephone"); const emailInput = document.getElementById("email");
/**
- VALIDATORS
- */
// Can only contain letters a-z in lowercase function isValidUsername(username) { return/^[a-z]+$/.test(username); }
// Must contain a lowercase, uppercase letter and a number function isValidPassword(password) { return/[a-z]/.test(password) && /[A-Z]/.test(password) && /[0-9]/.test(password) && /^(?=.\d)(?=.[a-z])(?=.[A-Z]).$/.test(password); }
// The telephone number must be in the format of (555) 555-5555 function isValidTelephone(telephone) { return /^(\d{3})\s{3}-\d{4}$/.test(telephone); }
// Must be a valid email address function isValidEmail(email) {}
/**
- FORMATTING FUNCTIONS
- */
function formatTelephone(text) {}
/**
- SET UP EVENTS
- */
function showOrHideTip(show, element) { // show element when show is true, hide when false if (show) { element.style.display = "inherit"; } else { element.style.display = "none"; } }
function createListener(validator) { return e => { const text = e.target.value; const valid = validator(text); const showTip = text !== "" && !valid; const tooltip = e.target.nextElementSibling; showOrHideTip(showTip, tooltip); }; }
usernameInput.addEventListener("input", createListener(isValidUsername));
passwordInput.addEventListener("input", createListener(isValidPassword));
telephoneInput.addEventListener("input", createListener(isValidTelephone));
emailInput.addEventListener("input", createListener(isValidEmail));
olushola oludipe
Web Development Techdegree Graduate 18,495 Pointsolushola oludipe
Web Development Techdegree Graduate 18,495 PointsHi Karl, In your code you have
^(\d{3})\s{3}-\d{4}$/.test(telephone); }
1.now if you check the first part of your regex you have
^(\d{3})
which is meant to catch (555), you forgot to escape the(
. to escape a special character you put\
before the character not after, so it's meant to be like this/^\(
. 1b.you made the same mistake after\d{3}
. so it's meant to be like this/^\(\d{3}\)\s
. this covers the "(555) "of the search2.you typed
\s{3}
which means "there white spaces". to fix this add a\d
before the{3}
. so that part will look like this\s\d{3}-
putting everything together the regex should look like this/^\(\d{3}\)\s\d{3}-\d{4}$/.test(telephone)
. its easy to mistype expression , so thats why it's a good idea to test them on sites like https://www.regexpal.com/ just to make sure they work properly . hope this helps, Good luck