Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial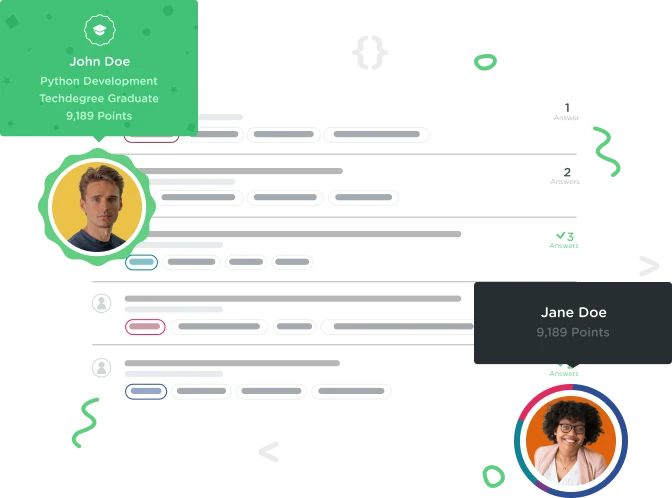
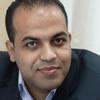
Ahmed Abdelhay
6,025 Pointsit fails always in same test case "Create a taco and redirect to the home page"
it fails always in same test case "Create a taco and redirect to the home page"
from flask import Flask, g, render_template, flash, redirect, url_for
from flask.ext.bcrypt import check_password_hash
from flask.ext.login import (LoginManager, login_user,
logout_user, login_required, current_user)
import forms
import models
DEBUG = True
PORT = 8000
HOST = '0.0.0.0'
app = Flask(__name__)
app.secret_key = 'top_secret'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
@login_manager.user_loader
def load_user(userid):
try:
return models.User.get(models.User.id == userid)
except models.DoesNotExist:
return None
@app.before_request
def before_request():
"""Connect to the database before each request."""
g.db = models.DATABASE
g.db.connect()
g.user = current_user
@app.after_request
def after_request(response):
"""Close the database connection after each request."""
g.db.close
return response
@app.route('/register', methods=('GET', 'POST'))
def register():
form = forms.RegisterForm()
if form.validate_on_submit():
flash("Yay, you registered!", "success")
models.User.create_user(
email=form.email.data,
password=form.password.data
)
return redirect(url_for('index'))
return render_template('register.html', form=form)
@app.route('/login', methods=('GET', 'POST'))
def login():
form = forms.LoginForm()
if form.validate_on_submit():
try:
user = models.User.get(models.User.email == form.email.data)
except models.DoesNotExist:
flash("Email is incompatible", "error")
else:
if check_password_hash(user.password, form.password.data):
login_user(user)
flash("You are logged in", "success")
return redirect(url_for('index'))
else:
flash("Password does not match", "error")
return render_template('login.html', form=form)
@app.route('/logout')
@login_required
def logout():
logout_user()
flash("You've been logged out! Come back soon!", "success")
return redirect(url_for('index'))
@app.route('/new_taco', methods=('GET', 'POST'))
@login_required
def taco():
form = forms.TacoForm()
if form.validate_on_submit():
models.Taco.create(user=g.user._get_current_object(),
protein=form.protein.data,
shell=form.shell.data,
cheese=form.cheese.data,
extras=form.extras.data.strip()
)
flash("Your taco has been added!!", "success")
return redirect(url_for('index'))
return render_template('taco.html', form=form)
@app.route('/')
def index():
tacos = models.Taco.select().limit(10)
return render_template('index.html', tacos=tacos)
if __name__ == '__main__':
models.initialize()
try:
with models.DATABASE.transaction():
models.User.create_user(
email='a@a.com',
password='123123'
)
except ValueError:
pass
import datetime
from flask.ext.bcrypt import generate_password_hash
from flask.ext.login import UserMixin
from peewee import *
DATABASE = SqliteDatabase('taco.db')
class User(UserMixin, Model):
email = CharField(unique=True)
password = CharField(max_length=100)
class Meta:
database = DATABASE
def get_tacos(self):
return Taco.select().where(Taco.user == self)
def get_stream(self):
return Taco.select().where(
(Taco.user == self)
)
@classmethod
def create_user(cls, email, password):
try:
cls.create(
email=email,
password=generate_password_hash(password))
except IntegrityError:
raise ValueError("User already exists")
class Taco(Model):
protein = CharField()
shell = CharField()
cheese = BooleanField(default=True)
extras = TextField()
user = ForeignKeyField(
rel_model=User,
related_name='tacos'
)
class Meta:
database = DATABASE
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, Taco], safe=True)
DATABASE.close()
from flask_wtf import Form
from wtforms import StringField, PasswordField, BooleanField, TextAreaField
from wtforms.validators import (DataRequired, ValidationError, Email,
Length, EqualTo)
from models import User
def email_exists(form, field):
if User.select().where(User.email == field.data).exists():
raise ValidationError('User with that email already exists')
class RegisterForm(Form):
email = StringField(
'Email',
validators=[
DataRequired(),
Email(),
email_exists,
])
password = PasswordField(
'Password',
validators=[
DataRequired(),
Length(min=2),
EqualTo('password2', message='Passwords must match')
])
password2 = PasswordField(
'Confirm Password',
validators=[DataRequired()]
)
class LoginForm(Form):
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('Password', validators=[DataRequired()])
class TacoForm(Form):
protein = StringField('Protein')
shell = StringField('Shell', validators=[DataRequired()])
cheese = BooleanField('Cheese')
extras = TextAreaField("Any extras?")
<!doctype html>
<html>
<head>
<title>Tacocat</title>
<link rel="stylesheet" href="/static/css/normalize.css">
<link rel="stylesheet" href="/static/css/skeleton.css">
<link rel="stylesheet" href="/static/css/tacocat.css">
</head>
<body>
{% with messages=get_flashed_messages() %}
{% if messages %}
<div class="messages">
{% for message in messages %}
<div class="message">
{{ message }}
</div>
{% endfor %}
</div>
{% endif %}
{% endwith %}
<div class="container">
<div class="row">
<div class="u-full-width">
<nav class="menu">
<!-- menu goes here -->
{% if current_user.is_authenticated() %}
<a href="{{ url_for('logout') }}" title="Log Out">Log out</a>
<a href="{{ url_for('taco') }}" title="New Taco">Add a New Taco</a>
{% else %}
<a href="{{ url_for('login') }}" title="Log in">Log in</a>
<a href="{{ url_for('register') }}" title="Sign Up">Sign Up</a>
{% endif %}
</nav>
{% block content %}{% endblock %}
</div>
</div>
<footer>
<p>An MVP web app made with Flask on <a href="http://teamtreehouse.com">Treehouse</a>.</p>
</footer>
</div>
</body>
</html>
{% extends 'layout.html' %}
{% block content %}
<h2>Tacos</h2>
{% if tacos.count() %}
<table class="u-full-width">
<thead>
<tr>
<th>Protein</th>
<th>Cheese?</th>
<th>Shell</th>
<th>Extras</th>
</tr>
</thead>
<tbody>
{% for taco in tacos %}
<!-- taco attributes here -->
<!-- your not outputting any attributes try the below -->
<tr>{{taco.protein}}</tr>
<tr>{{taco.cheese}}</tr>
<tr>{{taco.shell}}</tr>
<tr>{{taco.extras}}</tr>
{% endfor %}
</tbody>
</table>
{% else %}
<!-- message for missing tacos -->
<p>no tacos yet</p>
{% endif %}
{% endblock %}
1 Answer
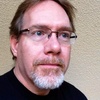
Chris Freeman
Treehouse Moderator 68,423 PointsYou are SO close! The route for a taco should be '/taco'
not '/new_taco'
. With this change you should pass the challenge. Congratulations!!
Post back if you need any more help. Good luck!!