Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial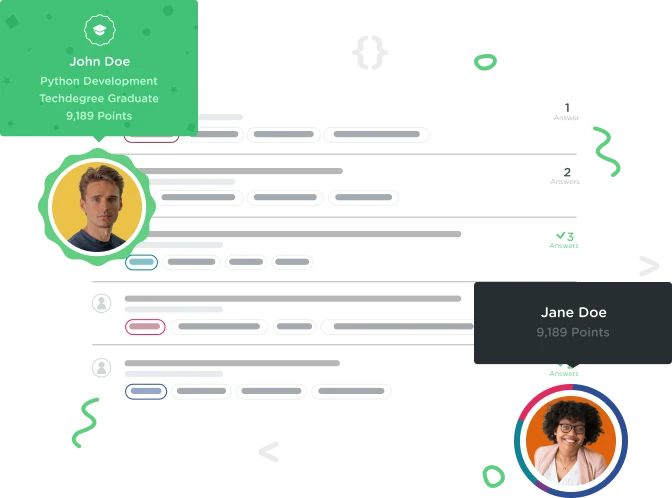

Juneau Lim
13,362 PointsIt has been 3 days I tried this. I'm desperate. Please help me with promptForWord().
I think I've spent more than 5 hours on this, but I can't make it work. Please help me.
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public List<String> run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(results);
return results;
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
System.out.println(phrase + ": ");
String result;
Boolean ok = false;
do {
result = mReader.readLine();
for (String badWord : mCensoredWords) {
if (result.contains(badWord)){
ok = false;
System.out.println("Enter again!");
continue;
} else {
Pattern pattern = Pattern.compile("__([^__]+)__");
Matcher matcher = pattern.matcher(result);
if (matcher.find()) {
String[] argsArr = result.split(", ");
List<String> arrsList = new ArrayList<>();
for (int i = 1; i < argsArr.length; i++){
arrsList.add(argsArr[i]);
}
Template template = new Template(result);
Prompter prompter = new Prompter();
Template tmpl = new Template(result);
result = tmpl.render(arrsList);
}
ok = true;
}
}
} while (!ok);
return result;
}
}
1 Answer

adrian miranda
13,561 PointsThere are a number of issues in this, it might be useful to back up and try again. One thing that jumps out is the continue after you check for a bad word. The continue will only restart the inner loop, the for loop. As a result, won't do another readline() and will just check the same word again... Probably you want to set a value that tells you that you found a bad word, and break out of the loop. A bigger issue is what you do when you don't match a badword (the else). You start looking for the user to enter a word surrounded in underscores. My recollection is that the user at this point should be entering an actual noun or adjective. Why would they be entering a word with underscores? I think you just want to loop through the badwords, and if you find one that matches the word they entered. If they entered a bad word, prompt for another word, otherwise return the word. It's really relatively simple. There is no need to do any pattern matching in this file. The pattern matching goes in Template.java, at least that's how I recall it.
Juneau Lim
13,362 PointsJuneau Lim
13,362 PointsThank you for the detailed answer! I used Reg because first I didn't, I saw that the test case, a noun was actually
__this__ form. which is __super__ weird.
Maybe it will be better to start from scratch.