Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial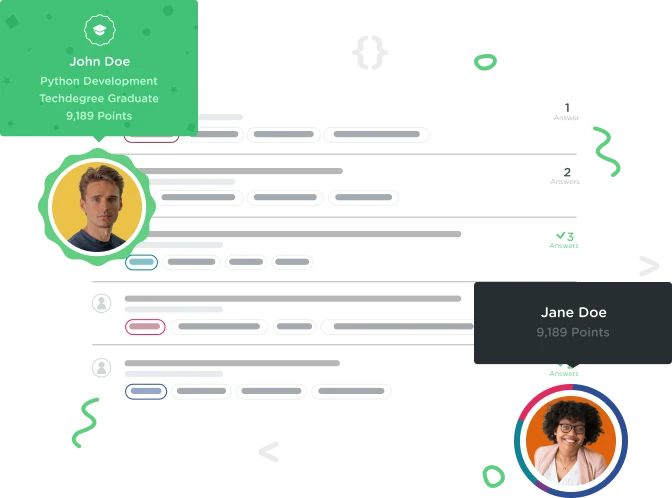

Roshan Kashif
2,976 PointsIt is giving me an error saying"key array points to a video with title"
what does the error mean
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.List;
import java.util.TreeMap;
public class QuickFix {
List<Video> videoList;
Map<String, Video> videoMap;
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video videoToAdd = new Video("The Beginning Bits");
// TODO(2): Add to the course videos as the second video.
videoList = course.getVideos();
videoList.add(1, videoToAdd);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> newVideoMap = videosByTitle(course);
Video video = newVideoMap.get(oldTitle);
videoMap.put(video.getTitle(), video);
}
public Map<String, Video> videosByTitle(Course course) {
videoList = course.getVideos();
Map<String, Video> videoMap = new TreeMap<>();
for (Video video : videoList) {
videoMap.put(video.getTitle(), videoList.get(1));
}
return videoMap;
}
}
1 Answer

Dan Johnson
40,532 PointsIn fixVideoTitle you want to set the new title on the Video object (using setTitle) that you got from the map using the old title, rather than modifying the map. You don't have to deal with instance variables for this challenge.
Roshan Kashif
2,976 PointsRoshan Kashif
2,976 Pointsvideo.setTitle(newTitle); Is that what you mean
Dan Johnson
40,532 PointsDan Johnson
40,532 PointsYep, that'll update the title.
Roshan Kashif
2,976 PointsRoshan Kashif
2,976 PointsI did that but its still giving me the same error
Dan Johnson
40,532 PointsDan Johnson
40,532 PointsIn your videosByTitle method you need to map to the Video you're getting the title from.
Roshan Kashif
2,976 PointsRoshan Kashif
2,976 PointsI still can't get this to work
Dan Johnson
40,532 PointsDan Johnson
40,532 PointsUsing variables local to the methods rather than instance variables might help your sort through it easier. I did check it using instance variables and it'll still pass but it makes it harder to follow.
If that still doesn't help you can post your updated code and I'll look it over again.