Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial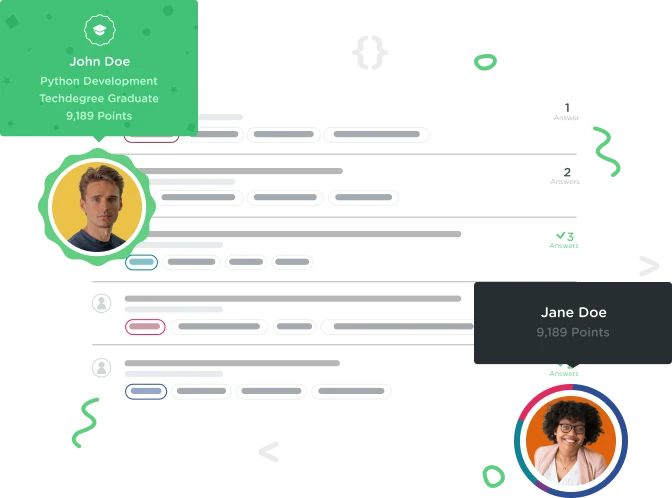

India Poetzscher
Courses Plus Student 3,821 PointsIt keeps saying cannot convert value of type 'Double.Type' to expected argument type 'Double' and same for 'String'!
In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values.
For this task we want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location.
In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = String()
self.location = Location(latitude: Double, longitude: Double)
}
let someBusiness = Business(name: String, location: Location)
}
1 Answer

walim
iOS Development with Swift Techdegree Student 1,868 PointsHi,
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
var location: Location = Location(latitude: 20, longitude: 20)
// init properties into init function makes Swift ask you for a initial value when you instantiate an object from that class
init(name: String) {
self.name = name
}
}
let someBusiness = Business(name: "McDonalds'")
someBusiness.location = Location(latitude: 30, longitude: 30) // See value and reference types
print(someBusiness.name) // Prints McDonalds'
print(someBusiness.location) // Prints Location(latitude: 30.0, longitude: 30.0)