Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial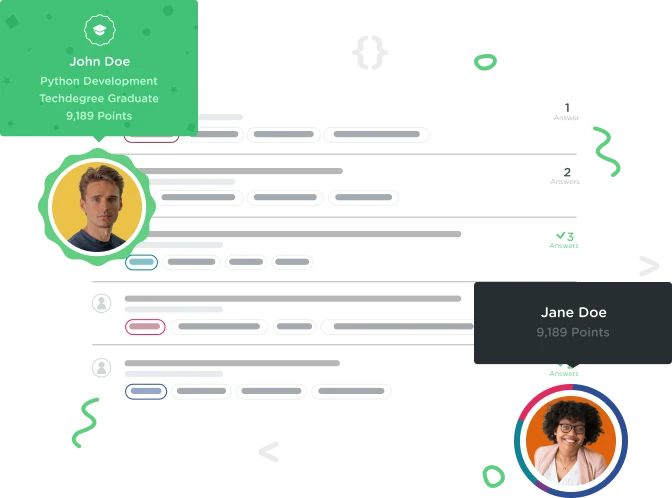

Jake barnly
177 PointsIt keeps saying my camera can't access external storage OR it says the camera is working. HELP!!!!!!!!!
It doesn't say that there is any errors that I see but it keeps shutting off or it will work 10% of the time.
package com.Jellybean.jellybean;
import java.io.File; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Locale;
import android.app.ActionBar; import android.app.AlertDialog; import android.app.FragmentTransaction; import android.content.DialogInterface; import android.content.Intent; import android.net.Uri; import android.os.Bundle; import android.os.Environment; import android.provider.MediaStore; import android.support.v13.app.FragmentPagerAdapter; import android.support.v4.app.FragmentActivity; import android.support.v4.view.ViewPager; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.view.Window; import android.widget.Toast;
import com.parse.ParseAnalytics; import com.parse.ParseUser;
public class MainActivity extends FragmentActivity implements ActionBar.TabListener {
public static final String TAG = MainActivity.class.getSimpleName();
public static final int TAKE_PHOTO_REQUEST = 0;
public static final int TAKE_VIDEO_REQUEST = 1;
public static final int PICK_PHOTO_REQUEST = 2;
public static final int PICK_VIDEO_REQUEST = 3;
public static final int MEDIA_TYPE_IMAGE = 4;
public static final int MEDIA_TYPE_VIDEO = 5;
protected Uri mMediaUri;
protected DialogInterface.OnClickListener mDialogListner = new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
switch(which){
case 0: //Take a picture
Intent takePhotoIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_IMAGE);
if(mMediaUri == null){
//display and error
Toast.makeText(MainActivity.this, R.string.error_external_storage, Toast.LENGTH_LONG).show();
}
else{
takePhotoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
startActivityForResult(takePhotoIntent, TAKE_PHOTO_REQUEST);
}
break;
case 1: //Take video
break;
case 2: // Choose a Picture
break;
case 3: // Choose Video
break;
}
}
private Uri getOutputMediaFileUri(int mediaType) {
// To be safe, you should check that the SDCard is mounted
// using Environment.getExternalStorageState() before doing this.
if(isExternalStorageAvailable()){
//get the URI
//1. Get the external storage
String appName = MainActivity.this.getString(R.string.app_name);
File mediaStorageDir = new File(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES),
appName);
//2. Create our subdirectory
if(! mediaStorageDir.exists()){
if(! mediaStorageDir.mkdirs());
Log.e(TAG, "Failed to create directory.");
return null;
}
//3. Create a file name
//4. Create the file
File mediaFile;
Date now = new Date();
String timestamp = new SimpleDateFormat("yyyyMMdd_HHmmss", Locale.US).format(now);
String path = mediaStorageDir.getPath() + File.separator;
if(mediaType == MEDIA_TYPE_IMAGE){
mediaFile = new File(path + "IMG_" + timestamp + ".jpg");
}
else if(mediaType == MEDIA_TYPE_VIDEO){
mediaFile = new File(path + "VID_" + timestamp + ".mp4");
}
else{
return null;
}
Log.d(TAG,"File: " + Uri.fromFile(mediaFile));
//5. Return the file's URI
return Uri.fromFile(mediaFile);
}
else{
return null;
}
}
private boolean isExternalStorageAvailable(){
String state = Environment.getExternalStorageState();
if(state.equals(Environment.MEDIA_MOUNTED)){
return true;
}
else{
return false;
}
}
};
/**
* The {@link android.support.v4.view.PagerAdapter} that will provide
* fragments for each of the sections. We use a {@link FragmentPagerAdapter}
* derivative, which will keep every loaded fragment in memory. If this
* becomes too memory intensive, it may be best to switch to a
* {@link android.support.v13.app.FragmentStatePagerAdapter}.
*/
SectionsPagerAdapter mSectionsPagerAdapter;
/**
* The {@link ViewPager} that will host the section contents.
*/
ViewPager mViewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_main);
ParseAnalytics.trackAppOpened(getIntent());
ParseUser currentUser = ParseUser.getCurrentUser();
if(currentUser == null){
navigateToLogin();
}
else{
Log.i(TAG, currentUser.getUsername());
}
// Set up the action bar.
final ActionBar actionBar = getActionBar();
actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
// Create the adapter that will return a fragment for each of the three
// primary sections of the activity.
mSectionsPagerAdapter = new SectionsPagerAdapter(this,getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.pager);
mViewPager.setAdapter(mSectionsPagerAdapter);
// When swiping between different sections, select the corresponding
// tab. We can also use ActionBar.Tab#select() to do this if we have
// a reference to the Tab.
mViewPager
.setOnPageChangeListener(new ViewPager.SimpleOnPageChangeListener() {
@Override
public void onPageSelected(int position) {
actionBar.setSelectedNavigationItem(position);
}
});
// For each of the sections in the app, add a tab to the action bar.
for (int i = 0; i < mSectionsPagerAdapter.getCount(); i++) {
// Create a tab with text corresponding to the page title defined by
// the adapter. Also specify this Activity object, which implements
// the TabListener interface, as the callback (listener) for when
// this tab is selected.
actionBar.addTab(actionBar.newTab()
.setText(mSectionsPagerAdapter.getPageTitle(i))
.setTabListener(this));
}
}
private void navigateToLogin() {
//if there is no current user, execute these lines. (Which takes them to the login screen)
Intent intent = new Intent(this, LoginActivity.class);
intent.addFlags(intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int itemId = item.getItemId();
switch(itemId){
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
case R.id.action_edit_friends:
Intent intent = new Intent(this, EditFriendsActivity.class);
startActivity(intent);
case R.id.action_camera:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setItems(R.array.camera_choices, mDialogListner );
AlertDialog dialog = builder.create();
dialog.show();
}
if (itemId == R.id.action_logout) {
ParseUser.logOut();
navigateToLogin();
return true;
}
else if(itemId ==R.id.action_edit_friends ){
Intent intent = new Intent(this, EditFriendsActivity.class);
startActivity(intent);
}
return super.onOptionsItemSelected(item);
}
@Override
public void onTabSelected(ActionBar.Tab tab,
FragmentTransaction fragmentTransaction) {
// When the given tab is selected, switch to the corresponding page in
// the ViewPager.
mViewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(ActionBar.Tab tab,
FragmentTransaction fragmentTransaction) {
}
@Override
public void onTabReselected(ActionBar.Tab tab,
FragmentTransaction fragmentTransaction) {
}
}
3 Answers

Danial Goodwin
13,247 PointsOne way to get this error is by not having the WRITE_EXTERNAL_STORAGE permission. So, please check that you have the following line in the AndroidManifest.xml:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />

Andre Colares
5,437 PointsHere is the functional code (ANDROID STUDIO 1.4):
protected DialogInterface.OnClickListener mDialogListener =
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
switch (which){
case 0: //Take Picture
Intent takePhotoIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// get path to save images
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_IMAGE);
if(mMediaUri==null){
//display an error
Toast.makeText(MainActivity.this,
R.string.error_external_storage,
Toast.LENGTH_LONG).show();
}else {
takePhotoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
startActivityForResult(takePhotoIntent, TAKE_PHOTO_REQUEST);
}
break;
case 1: //Take Video
break;
case 2: //Choose Picture
break;
case 3: //Choose Video
break;
}
}
private Uri getOutputMediaFileUri(int mediaType) {
//To be safe, you should check that the SDCard is mounted
//using Environment.getExternalStorageState() before doing this
if(isExternalStorageAvaible()){
//Get the URI
//1. Get the external storage directory
String appName = MainActivity.this.getString(R.string.app_name);
File mediaStorageDir = new File(
Environment.getExternalStoragePublicDirectory(
Environment.DIRECTORY_PICTURES),
getString(R.string.app_name)
);
// create subdirectory
if ( ! mediaStorageDir.exists() ) {
if ( ! mediaStorageDir.mkdirs() ) {
Log.e(TAG, "Failed to create directory");
return null;
}
}
//3.Create a file name
File mediaFile;
//4. Create the file
Date now = new Date();
String timestamp = new SimpleDateFormat("yyyyMMdd_HHmmss", Locale.US).format(now);
String path = mediaStorageDir.getPath() + File.separator;
switch (mediaType) {
case MEDIA_TYPE_IMAGE:
mediaFile = new File(path + "IMG_" + timestamp + ".jpg");
break;
case MEDIA_TYPE_VIDEO:
mediaFile = new File(path + "VID_" + timestamp + ".mp4");
break;
default:
return null;
}
Log.d(TAG, "File: " + Uri.fromFile(mediaFile));
//5. Return the file's Uri
return Uri.fromFile(mediaFile);
}else {
return null;
}
}
private boolean isExternalStorageAvaible(){
String state = Environment.getExternalStorageState();
return state.equals(Environment.MEDIA_MOUNTED) ? true : false;
}
};

Andre Colares
5,437 PointsHere is the ANDROID MANIFEST (ANDROID STUDIO 1.4)
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.andrecolares.ribbit" >
<!-- To access Google+ APIs: -->
<uses-permission android:name="android.permission.INTERNET" />
<!--
To retrieve OAuth 2.0 tokens or invalidate tokens to disconnect a user. This disconnect option is required to comply with the Google+ Sign-In developer policies --> <uses-permission android:name="android.permission.USE_CREDENTIALS" /> <!-- To retrieve the account name (email) as part of sign-in: --> <uses-permission android:name="android.permission.GET_ACCOUNTS" /> <!-- To auto-complete the email text field in the login form with the user's emails --> <uses-permission android:name="android.permission.READ_PROFILE" /> <uses-permission android:name="android.permission.READ_CONTACTS" /> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <uses-permission android:name="android.permission.CAMERA" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-feature android:name="android.hardware.camera" android:required="true" />
<application
android:name=".RibbitApplication"
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name"
android:screenOrientation="portrait" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:name=".LoginActivity"
android:label="@string/title_activity_login"
android:screenOrientation="portrait" >
</activity>
<activity
android:name=".SignUpActivity"
android:label="@string/title_activity_sign_up"
android:parentActivityName=".LoginActivity"
android:screenOrientation="portrait" >
</activity>
<meta-data
android:name="com.google.android.gms.version"
android:value="@integer/google_play_services_version" />
<activity
android:name=".EditFriendsActivity"
android:label="@string/title_activity_edit_friends"
android:parentActivityName=".MainActivity"
android:screenOrientation="portrait">
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value="com.andrecolares.ribbit.MainActivity" />
</activity>
</application>
</manifest>