Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial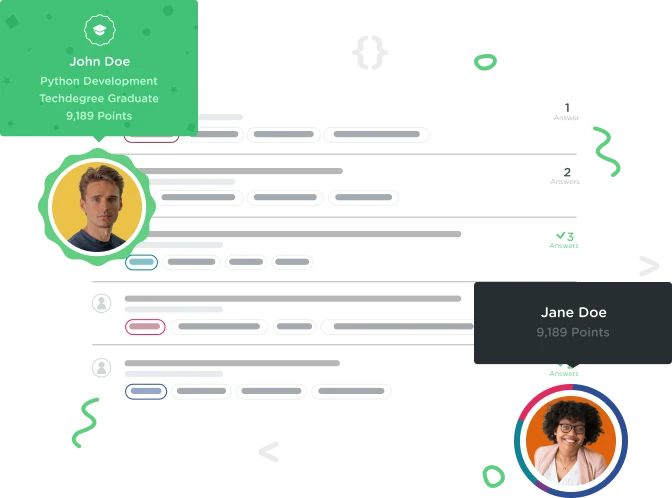

Ben Manson
5,882 PointsIt looks like my output is what they're asking for! What do I do?
Help!
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
var index = 0
while index < 1 {
println(numbers)
index++
}
3 Answers

Dan Johnson
40,532 PointsThe output looked correct since you ended up printing the entire list once instead of each element on its own. Here's one solution:
// There are ten elements in the array, so we want the range 0-9.
// We can have the count property get this length for us so we
// don't have to worry about updating it or making a mistake.
while index < numbers.count {
// Grab the element at the current index.
// Passing just numbers in will print the entire
// array.
println(numbers[index])
index++
}

Ben Manson
5,882 Pointsso the index is a variable containing an integer... we initially assign it the value 0, but as it runs through the loop the value increases by one each time the loop runs, until it reaches the value specified by numbers.count, whose value is determined by the number of items in the array. Then the loop terminates. Correct?
Oh yeah! Dan, also: I see that the value of index only increases by 1 each time the loop goes through because of the index++ command, which is why loop terminates after it runs 10 times (10 being the value of numbers.count).
So why does adding [index] within the parenthesis have the loop print only individual items within the array rather than the whole array?
Thanks for your help with you first response!

Dan Johnson
40,532 Pointsnumbers refers to the array itself, the collection of elements. numbers[index] refers to a specific element contained within that collection at position index.

Ben Manson
5,882 PointsOh! I think I get it now!
so the first time the loop runs, the value of index is 0, so it'll print the first time in the first time of the index (it's as if the square brackets said [0]), the second time the value of index will be 1, so it'll print the second item (it's as if the square said said [1]), and so on until the loop terminates. That's very cool!
Thanks, Dan!

Kris B
11,214 PointsYour code would print 1 and stop. This is because of the "index < 1". You're saying print out the indexes less than 1. They're asking for 1 - 10. The below code will complete the task:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
var index = 1
while index <= 10 {
println(index)
index++
}
Remember the logical operator < just means less than, <= means less than or equal to, so its required to print 10. You also need to set the index (counter) to 1 otherwise it prints 0 - 10, not 1 - 10.