Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial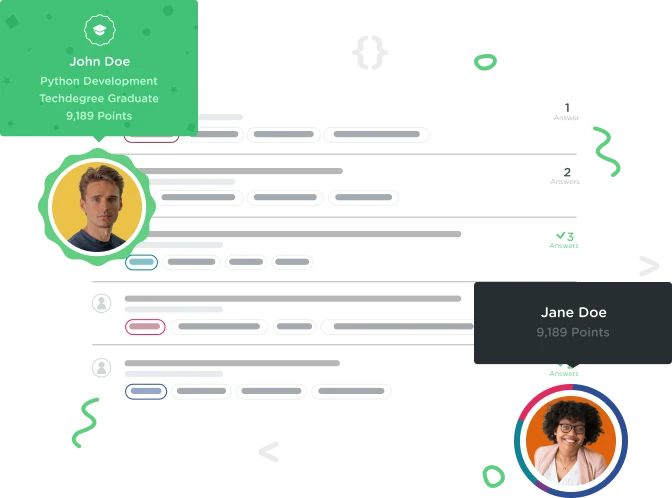

Amy Tomey
12,847 Pointsit looks ok... but it does not work.
here's my code...
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
Let correctGuess = 0;
// 2. Store the rank of a player
Let playerRank =;
// 3. Select the <main> HTML element
const main = document.querySelector = ('main');
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
const q1 = prompt('What is your favorite color?');
if(q1.toUpperCase() === 'GREEN') {
correctGuess = (correctGuess +1);
}
const q2 = prompt('What is your favorite main dish?');
if(q2.toUpperCase() === 'LASAGNA') {
correctGuess +=1;
}
const q3 = prompt('What is your favorite dessert?');
if(q3.toUpperCase() === 'STRAWBERRY SHORTCAKE') {
correctGuess +=1;
}
const q4 = prompt('What is your favorite hot beverage?');
if(q1.toUpperCase() === 'HOTCHOCOLATE') {
correctGuess +1;
}
const q5 = prompt('What are your favorite cookies?');
if(q1.toUpperCase() === 'SNICKERDOODLES') {
correctGuess +1= (correctGuess +1);
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if (correctGuess === 5) {
rank = "You win a GOLD Crown!"
} else if (correctGuess >=3) {
rank = "You win a SILVER Crown!"
} else if (correctGuess >=1) {
rank = "You win a BRONZE Crown!"
} else {
rank ="Sorry, You don't get a Crown."
}
// 6. Output results to the <main> element
main.innerHTML = `
<h2>You got ${correctGuess}out of 5 questions right. </h2>
<p> <strong> ${rank}</strong></p)
`;
Mod edit: added formatting for JavaScript code. Check out the "markdown cheatsheet" linked below the comment box for how to add code formatting and improve readability :)
1 Answer
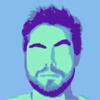
Cameron Childres
11,817 PointsHey Amy,
A good habit to get in to is checking the browser's JavaScript console for errors when your code is behaving unexpectedly. In Chrome on Windows you can open this with with shortcut CTRL+SHIFT+I and then make sure you're on the "console" tab. Here's what I see in the console when I run your code:
Uncaught SyntaxError: Unexpected identifier preview.js:6
This tips us off that something isn't written correctly in the file preview.js on line 6:
Let correctGuess = 0;
let
is case sensitive. Since it's capitalized it won't be recognized correctly which throws the browser off.
After correcting this on line 6 and 10, run your code again. The console will show another error:
Uncaught SyntaxError: Unexpected token ';' preview.js:10
The assignment for playerRank
is expecting a value but the right side is empty. You can set it to an empty string like so:
let playerRank = '';
From here see if you can work out the rest of the issues with your code. Paying attention to the errors in your console will help to find the location of problems quickly. I also recommend rewatching the video and comparing your code to the instructor's line by line, looking out for any differences. Remember that precision counts. Little typos can bring down a whole program! Feel free to let me know if you get totally stuck and I'll see if I can nudge you in the right direction.
Here's some hints on other things to look at in your code:
- q4 and q5 aren't checking against their respective answers
- q4 and q5 won't add anything to correctGuess
- the variable playerRank is never used
- check main.innerHTML for typos