Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial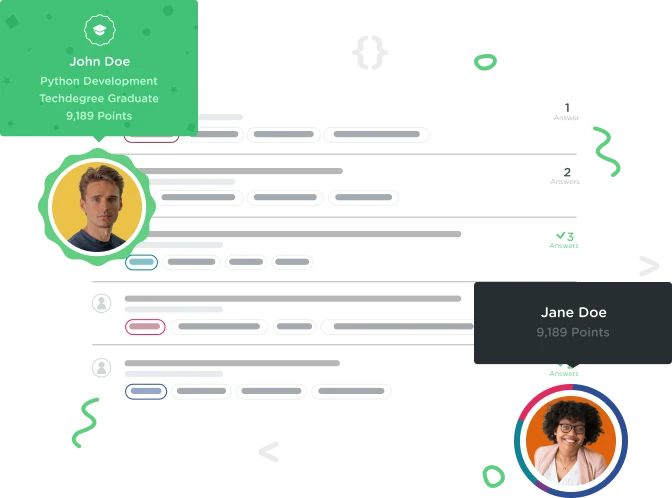

james white
78,399 PointsIt passes the final challenge for the course, but...
Here's the code that passed (after hours and hours of trial and error):
@Test
public void vendingOperationSuccessful() throws Exception {
assertEquals(0, machine.getRunningSalesTotal());
}
Don't have a clue as to WHY that was the correct answer, though.
In my way of thinking it seems to me that
a one line assert (added to the existing 'vendingWhenStockedReturnsItem()' test)
would have been more "right" somehow:
@Test
public void vendingWhenStockedReturnsItem() throws Exception {
machine.addMoney(75);
Item item = machine.vend("A1");
assertEquals("Twinkies", item.getName());
assertEquals(75, machine.getRunningSalesTotal());
}
But I guess I was just being stupid in using the following train of thought (tracing though what was initially given for the challenge):
runningSalesTotal = 0;
(see the the 'public VendingMachine' code inside 'VendingMachine.java')
runningSalesTotal += price;
(see the the 'public Item vend' code inside 'VendingMachine.java')
Once the 'runningSalesTotal' can be retrieved using this getter code (also from inside 'VendingMachine.java'):
public int getRunningSalesTotal() {
return runningSalesTotal;
}
Then use 'machine' in place of vending machine
(looking at the '@Before' lines of code):
machine = new VendingMachine(notifier, 10, 10, 10);
Then the 'asserts' (if true test) should be that machine.getRunningSalesTotal() == 75.
after the tested restock (sale) using this line of code in the @Before code section
(where the final argument represents the price paid):
machine.restock("A1", "Twinkies", 10, 30, 75);
At least that's the way it seems to me..but maybe I guess I just misunderstood everything...
Anyway...I got my points/badges for this course so I guess it doesn't matter
if I actually learned anything -- on to the next course for more points and badges
Hopefully, though, this forum thread/post saves some people a lot of random guessing..
2 Answers
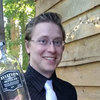
Evan Demaris
64,262 PointsHi james,
You actually did a really great job on this - and it sounds like you understand exactly what's going on with the code. Your posted answer:
@Test
public void vendingOperationSuccessful() throws Exception {
assertEquals(0, machine.getRunningSalesTotal());
}
Passes, because it's asserting that the running sales total will be some number. However, you're completely correct that the assert added to the vendingWhenStockedReturnsItem() is more "right". While your first answer is passing because it technically meets the requirements that the Challenge is checking against, the second answer is actually doing what the Challenge 'meant' for you to do. So really, a great answer would just be to add a new function that is identical to the vendingWhenStockedReturnsItem() function, but just adding your assert and changing the name. Adding the below code would pass the Challenge, and would be actually checking what it should:
@Test
public void vendingOperationSuccessful() throws Exception {
machine.addMoney(75);
Item item = machine.vend("A1");
assertEquals("Twinkies", item.getName());
assertEquals(75, machine.getRunningSalesTotal());
}
It might not feel like you're really advancing, because you keep pushing forward into areas that you aren't familiar with - you're challenging yourself, you are supposed to be having trouble working things out! But you really are learning as you go along. If you ever get completely stuck somewhere, remember that you aren't alone, we all get stuck sooner or later. Sometimes the best solution is to work your way through, sometimes it makes more sense to ask for help or see if someone else has already come up with a good way to do it. You shouldn't be afraid to ask for help here, too, because there are a lot of people who are happy to answer questions and help you learn.
The only bad coder is the one who gives up before they get good at it!
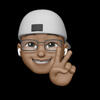
Allan Collado
10,248 Points@Test
public void vendingOperationSuccessful() throws Exception {
assertEquals(0, machine.getRunningSalesTotal());
}
Hi, I understand your code but what I don't understand
is why you are expecting 0!? for my understanding Im expecting a number bigger than the one in getRunningSalesTotal()?
Hannah Flynn
21,429 PointsHannah Flynn
21,429 PointsI need help. Can you show me the correct way you did code like you did with James, Evan?
Evan Demaris
64,262 PointsEvan Demaris
64,262 PointsHi Hannah, did you need help with this Challenge or with something else?