Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial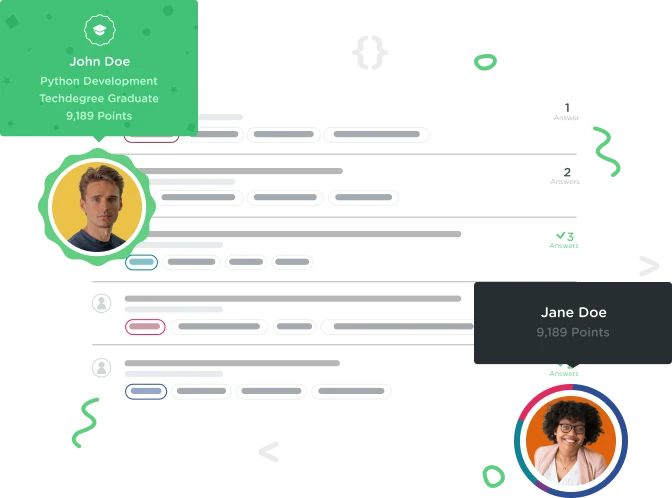

annikaheflin
577 PointsIt says that I have the wrong number of prints I am confused since I thought there is two.
What is wrong with my code? Also do you see anything else that's wrong.
import random
def even_odd(num):
start = 5
while start == True:
random.randint(1, 99)
start - 1
if num % 2 == 0:
print("{} is even").format(num)
else:
print("{} is odd").format(num)
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
1 Answer
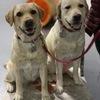
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Annika,
You do have a few bugs in your code.
First, and this should have been caught by the Challenge tester in task 2, is that you have declared your start variable inside your function, but the instructions tell you:
Make a new variable, outside of the
even_odd
function, namedstart
that's assigned to the number 5.
You're also getting a bit muddled by the rest of what code goes where. It's not totally obvious in the instructions but your code should comprise:
- import random (task 1); then
- a function called
even_odd
that returns whether the input value is odd or even (already created for you); then - setting a variable
start
to 5. (task 2); then lastly - a while loop that runs till
start
is falsey (task3)
You have a lot of code inside the even_odd function, but all the code you write should be outside that function.
Now for the specific items in your code:
Your while loop declaration has an error caused by a subtle difference between True
/False
and Truthy
/Falsey
. Basically, a value that is Truthy
acts like it is true, but isn't exactly true. The main difference is that if you explicitly ask a Truthy value if it is true, it will reply that it is not:
>>> 5 == True
False
But as long as you don't expressly compare it to True, truthy value will behave like True:
my_number = 1 # all non-zero numbers are 'Truthy'
if my_number:
print("my_number is acting like it is true!")
else:
print("my_number is acting like it is false!")
This will print "my_number is acting like it is true!"
. Now let's run the same code but with a falsey value:
my_number = 0 # all non-zero numbers are 'Truthy', zero is 'Falsey'
if my_number:
print("my_number is acting like it is true!")
else:
print("my_number is acting like it is false!")
Now we get "my_number is acting like it is false!"
.
In the above example, we used an if
statement, but the logic holds true for every other situation where we compare values. In your code, start
has a value of 5
, which is truthy, when it gets to 0, it will become falsey, so you can just write:
while start:
This will behave like true as long as start
isn't 0.
You're not doing anything with your random.randint()
call. You should be assigning that result to a temporary variable and then using that variable when you call the even_odd
function.
Next, you have a bug here:
start - 1
This just evaluates to 4 but doesn't actually do anything with that value. To change the value in the start
variable you need to assign the new value to start
. You can combine the assignment and deduction into a single operator: -=
.
Your use of the .format()
method is also not quite right. Remember that .format()
is a method on a string, therefore the .
should directly connect the string and the method. For example:
name = "Annika"
phrase = "hello, {}".format(name)
Also, print()
is a global function, so the parentheses should completely enclose the thing being printed.
print(phrase)
Is equivalent to:
print("hello, {}".format(name))
I know this sounds like a lot, but you are on the right track. Feel free to ask if you have any specific questions about the above.
Cheers
Alex