Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial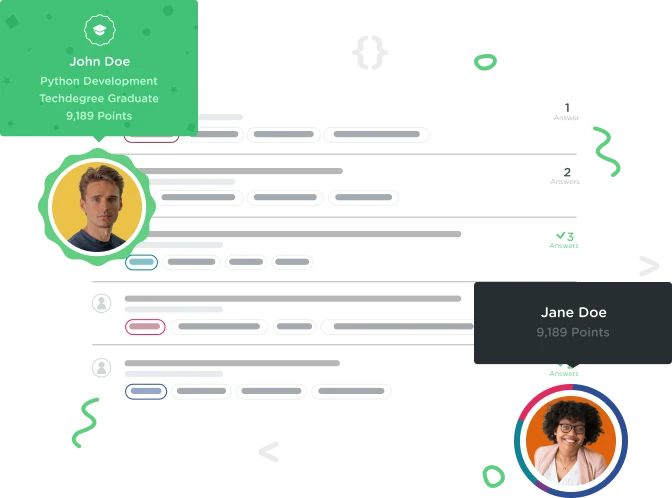

Kurt M
1,066 PointsIt seems that Swift 2 no longer uses #hash shorthand notation for external names of parameters of functions?
It seems that Swift 2 no longer uses #hash shorthand notation for external names of parameters of functions?
If this is true, what's now the best practice? Use the seemingly pedantic double-name syntax?
I guess I'm looking for (a) an overall explanation of internal v. external names that's updated for Swift 2 and (b) best practices.
2 Answers

Martin Wildfeuer
Courses Plus Student 11,071 PointsYou are right, Apple has tweaked the way function parameter names work in Swift 2. Have a look at Swift 2 Docs, the section is Function Paramter Names. It is explained in detail there, I will nevertheless give you a short overview.
What it comes down to:
- The first parameter of a function has no external parameter name by default
- All other parameters share the same external/internal parameter name by default
-
#
shorthand is no longer available, if you want the first parameter to have the same external name as the internal, you have to "double" the parameter names - All external parameter names can be overridden
- You can omit external parameter names by using
_
So, following the rules above:
// Please note that internalNameOne and internalNameTwo are the
// parameters accessible in the myFunction scope. That is, of course,
// the internal parameter names
// Standard behaviour
func myFunction(internalNameOne: Int, internalNameTwo: Int) {}
myFunction(1, internalNameTwo: 2)
// Second parameter with different external name
func myFunction(internalNameOne: Int, externalNameTwo internalNameTwo: Int) {}
myFunction(1, externalNameTwo: 2)
// This is how # used to work for the first parameter
func myFunction(internalNameOne internalNameOne: Int, externalNameTwo internalNameTwo: Int) {}
myFunction(internalNameOne: 1, externalNameTwo: 2)
// First and second parameters have different external parameter names
func myFunction(externalNameOne internalNameOne: Int, externalNameTwo internalNameTwo: Int) {}
myFunction(externalNameOne: 1, externalNameTwo: 2)
// Bonus: omitting external parameter names
func myFunction(internalNameOne: Int, _ internalNameTwo: Int) {}
myFunction(1, 2)
Note: You should try to follow Apple's example and hint to what parameter is expected in first place, wherever possible. That is the primary reason why no external name for the first parameter is default with Swift 2.
findMyString(string: String, language: String)
findMyString("Hello", language: "English")
Having said that, of course I wouldn't encourage anyone to force this style of naming, just use common sense ;)
// Naaaah...
func displayHintWithString(title: String) {}
// Better, bit long though
func displayHintWithTitle(title: String) {}
// Why not this way?
func displayHint(title title: String) {}
I hope this makes things clearer :)

Michael Benson
674 PointsLink: Swift 2.1 Documentation for anyone who needs it.
Kurt M
1,066 PointsKurt M
1,066 PointsThank you so much, Martin. Excellent response. I do appreciate both the link to docs (which I did not have) and the detailed explanation.
So it seems like typical best practice is to useโof courseโa descriptive function name and descriptive secondary parameter names?
Aside: For everybody's information, Apple just pushed out Xcode update to 7.0 which uses Swift 2.0 which does break some of the instruction given in this course: e.g. #hash shorthand notation or functions(?) like 'println' is now 'print' in the newer version of Xcode/Swift.