Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial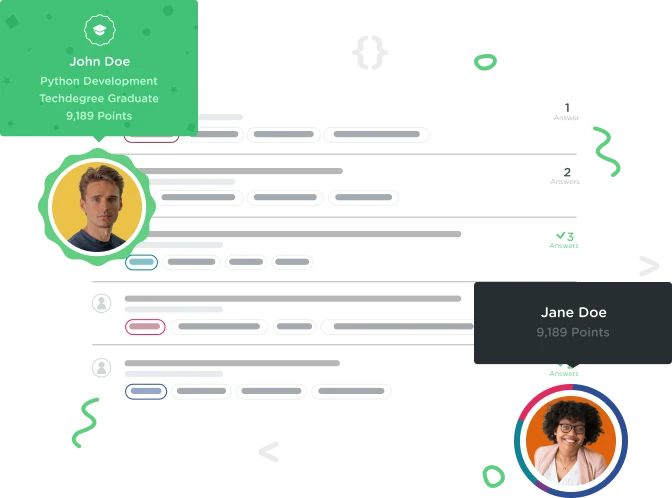

qlpxjevhuv
10,503 PointsIterables to List of Tuples
I'm having trouble with the code challenge below; I'm not sure how to get the first values of either iterables. Can someone please help?
#Create a function named combo() that takes two iterables and returns a list of tuples.
#Each tuple should hold the first item in each list, then the second set,
#then the third, and so on. Assume the iterables will be the same length.
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
def combo(iter1, iter2):
new_list = []
return new_list
Edit: Thanks for the help guys! I came up with this solution.
def combo(iter1, iter2):
new_list = []
for index, item in enumerate(iter1):
tup = item, iter2[index]
new_list.append(tup)
return new_list
3 Answers
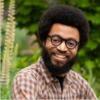
Nicolas Hampton
44,638 PointsTomasz,
The key here is to not make it any harder than it is. We're just looping through a list with a for loop, tracking the index as we go. We start with the index being 0, then loop through either of the arrays. Get the value of the array you're looping through, then use the index number you're tracking to get the value of the other list, then append both values to new_list as a tuple. There's nothing special we have to do to sync each list, we're just tracking the index as we loop through one list, so we can use that index to get the item from the other list. Just don't overthink it. Do what we always do when looping through a list and you should be fine. Good luck and happy coding.
Nicolas

Josh Keenan
19,652 PointsHere's my solution:
def combo(one, two):
return list(zip(one,two))
The zip function takes the Nth item from each item in the tuple, so thelist[0] with thestring[0], then thelist[1] and thestring[1] etc.. then using the list() function creates a list of these new zipped tuples!

Josh Keenan
19,652 PointsIf this doesn't work just post to this thread again!
Chris Grazioli
31,225 Pointsthis is awesome! one solid return statement... don't even have to make a variable... Hah!

Benedictt Vasquez
2,130 PointsThis works but is to long. :p
def combo (x, y):
list_x = list(x)
list_y = list(y)
tup = []
for index in list_x:
a = list_x.index(index)
c, h = list_x[a], list_y[a]
p = c, h
list(p)
tup.append(p)
return tup