Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial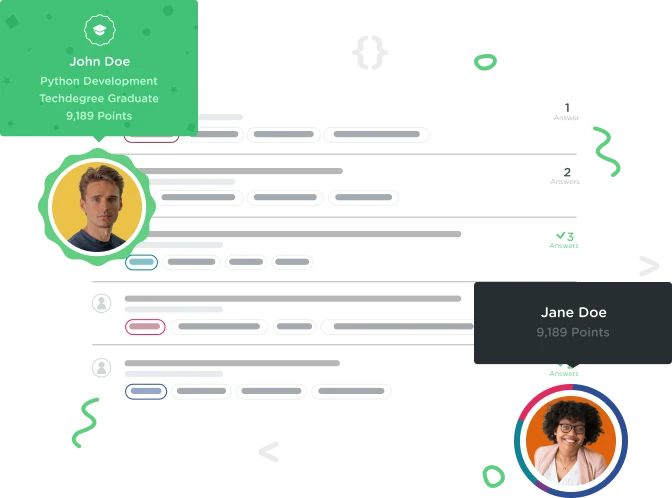

Courtney Schuman
1,469 PointsIterating through the list, every item that is not an integer is removed. And it semi works, but [1,2,3] is still there
I also tried for item in the_list: if type(item) is not int or is list: the_list.remove(item)
however when I do that it says that task 1 is not passing as if it removed the integers as well. feel like this is super easy, and I'm just over complicating it.
1 Answer
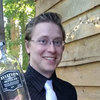
Evan Demaris
64,262 PointsHi Courtney,
There are a lot of ways to complete this task. It sounds like you're on part 2; you could just individually delete elements, like;
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0, the_list.pop(3))
# the_list = [1, "a", 2, 3, False, [1, 2, 3]]
del the_list[1]
# the_list = [1, 2, 3, False, [1, 2, 3]]
del the_list[3]
# the_list = [1, 2, 3, [1, 2, 3]]
del the_list[3]
# the_list = [1, 2, 3]
There are a few problems with your for
loop, though.
for item in the_list:
if type(item) is not int or is list:
the_list.remove(item)
For starters, you'd want to say !=
instead of is not
, and ==
instead of is
. Also, your 'or' statement is setup incorrectly; you'd need to say, if type(item) != int or type(item) == list
because otherwise you're checking if the type of the item isn't equal to int
, or if is list
. or
will run a completely separate check on the second statement independent of the first. However, that said, type
actually returns a type
object, not a string. Lastly though, when you iterate through a list in Python and remove objects, the list doesn't update in the way you might think. So what you're really doing is saying, check the item at index 0. Potentially, delete that item, making the list have a different item at index 0; the item which used to be at index 1. Next step, check the item which is now at index 1, which skips checking on the item now at index 0. If you want to iterate through a list and remove items as you go, it's probably better to make a new list with the same name as your original list, and have that list iterate through the original list and pick out just the items in it that you want to keep. However, there again, this is probably not ideal, because if you use a for
statement and compare types correctly, you'll find that boolean values are often considered equivalent to integers (False == 0, True == 1), so typechecking in general probably isn't the best way to solve this specific Challenge.